2020-11-10 01:49:29 -0600
| received badge | ● Famous Question
(source)
|
2020-09-16 16:26:10 -0600
| received badge | ● Popular Question
(source)
|
2020-04-03 07:49:22 -0600
| received badge | ● Notable Question
(source)
|
2019-12-31 13:28:00 -0600
| received badge | ● Popular Question
(source)
|
2019-04-24 17:04:46 -0600
| received badge | ● Notable Question
(source)
|
2018-12-01 08:17:39 -0600
| received badge | ● Popular Question
(source)
|
2018-07-12 22:23:52 -0600
| received badge | ● Popular Question
(source)
|
2018-06-08 11:56:48 -0600
| received badge | ● Famous Question
(source)
|
2017-08-01 06:09:13 -0600
| received badge | ● Notable Question
(source)
|
2017-06-02 04:38:23 -0600
| received badge | ● Famous Question
(source)
|
2017-04-22 14:53:26 -0600
| marked best answer | Efficient way to remove holes in an object Hello, I would like to remove holes in an object after I apply threshold function. Right now, I am applying dilate function a few times, then the erode function. It does okay, but in some cases, it changes the shape of the object. I also tried to use the MORPH_CLOSE, but I guess it is no good against big holes. I tried with different element size and shape but none of them helped. #include <opencv2/core/core.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/highgui/highgui.hpp>
using namespace cv;
using namespace std;
Mat src, srcGray, srcSmooth, srcThresh;
int morpho_elem = 1; // 0: MORPH_RECT, 1: MORPH_CROSS, 2: MORPH_ELLIPSE
int morpho_size = 3;
int morpho_qty = 9;
int const smooth_kernel = 11;
int main()
{
src = imread("source.png", 1);
cvtColor(src, srcGray, CV_BGR2GRAY);
for (int i = 1; i < smooth_kernel; i = i + 2)
{
medianBlur(srcGray, srcSmooth, i);
}
threshold(srcSmooth, srcThresh, 0, 255, CV_THRESH_BINARY | CV_THRESH_OTSU);
int dilation_type;
if (morpho_elem == 0){ dilation_type = MORPH_RECT; }
else if (morpho_elem == 1){ dilation_type = MORPH_CROSS; }
else if (morpho_elem == 2) { dilation_type = MORPH_ELLIPSE; }
Mat dilationDst, erosionDst;
Mat element = getStructuringElement(dilation_type,
Size(2 * morpho_size + 1, 2 * morpho_size + 1),
Point(morpho_size, morpho_size));
// Apply the dilation operation
dilate(srcThresh, dilationDst, element);
for (int i = 1; i < morpho_qty; i++)
{
dilate(dilationDst, dilationDst, element);
}
// Apply the erosion operation
erode(dilationDst, erosionDst, element);
for (int i = 1; i < morpho_qty; i++)
{
erode(erosionDst, erosionDst, element);
}
imshow("Final", erosionDst);
cvWaitKey(0);
return 0;
}
Source image: 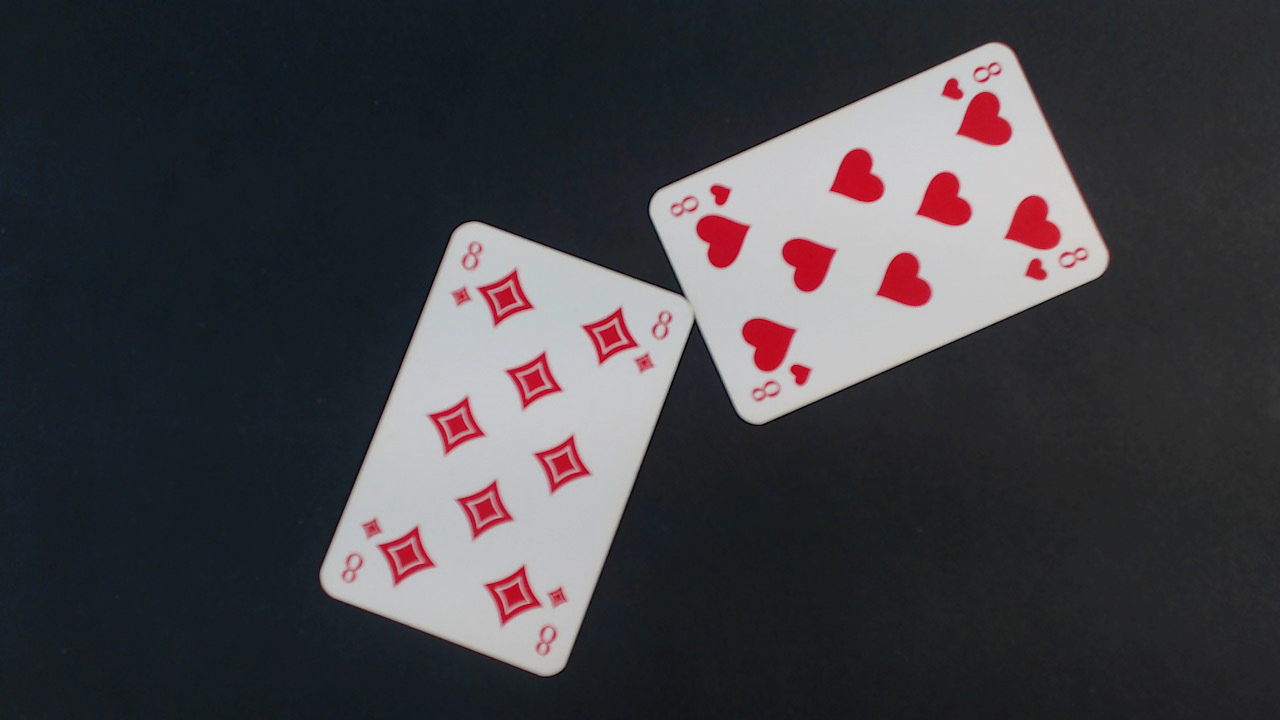 Binary image with holes:
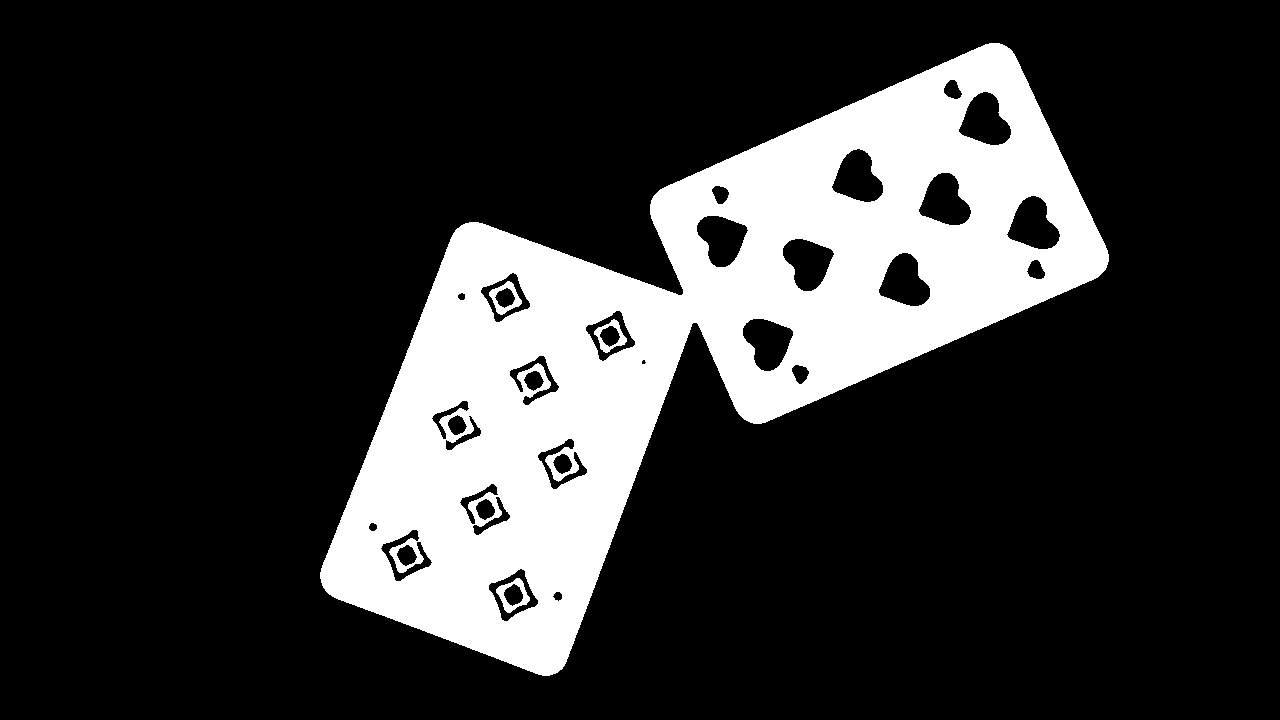 Output of my attempt:
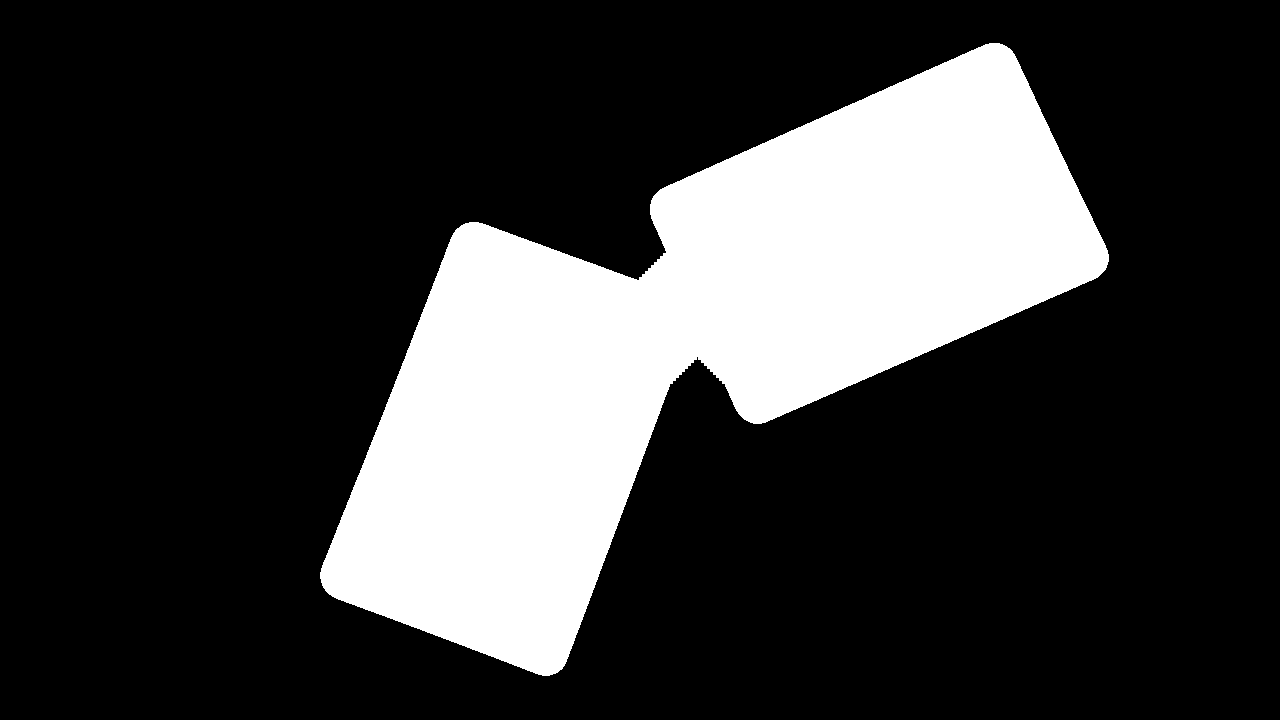 |
2017-04-04 19:06:28 -0600
| received badge | ● Famous Question
(source)
|
2017-03-18 19:05:07 -0600
| received badge | ● Popular Question
(source)
|
2017-03-10 02:41:33 -0600
| marked best answer | How to use approxPolyDP to close contours Hi, I would like to use approxPoly to close contours. But I am not quite sure about how it works. I am using OpenCV 2.4.11. So I have two images: 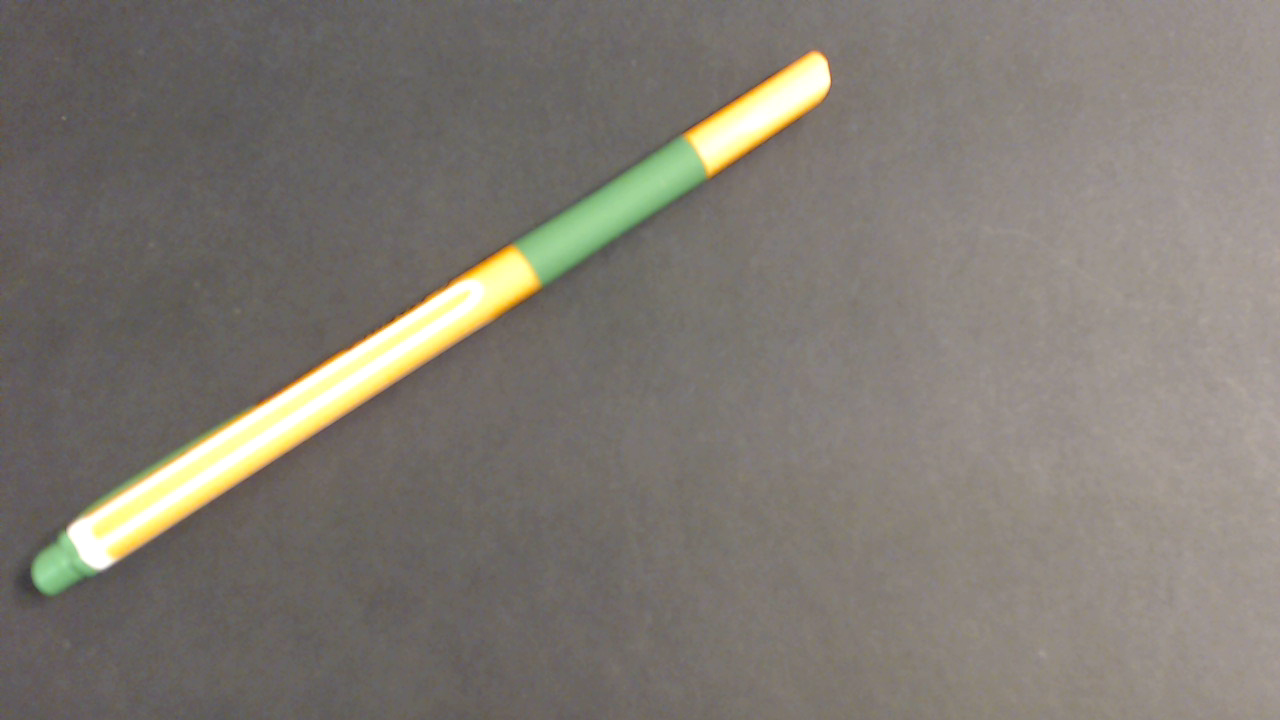 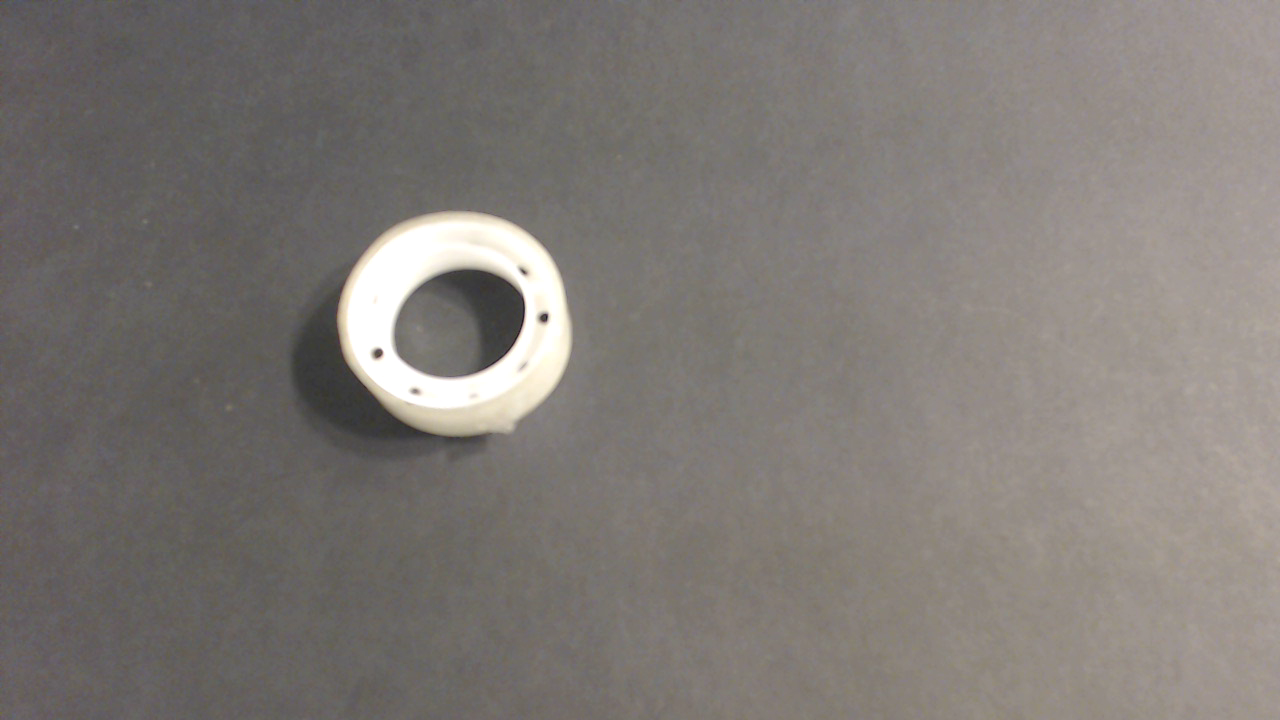 And I apply canny, then find contours. 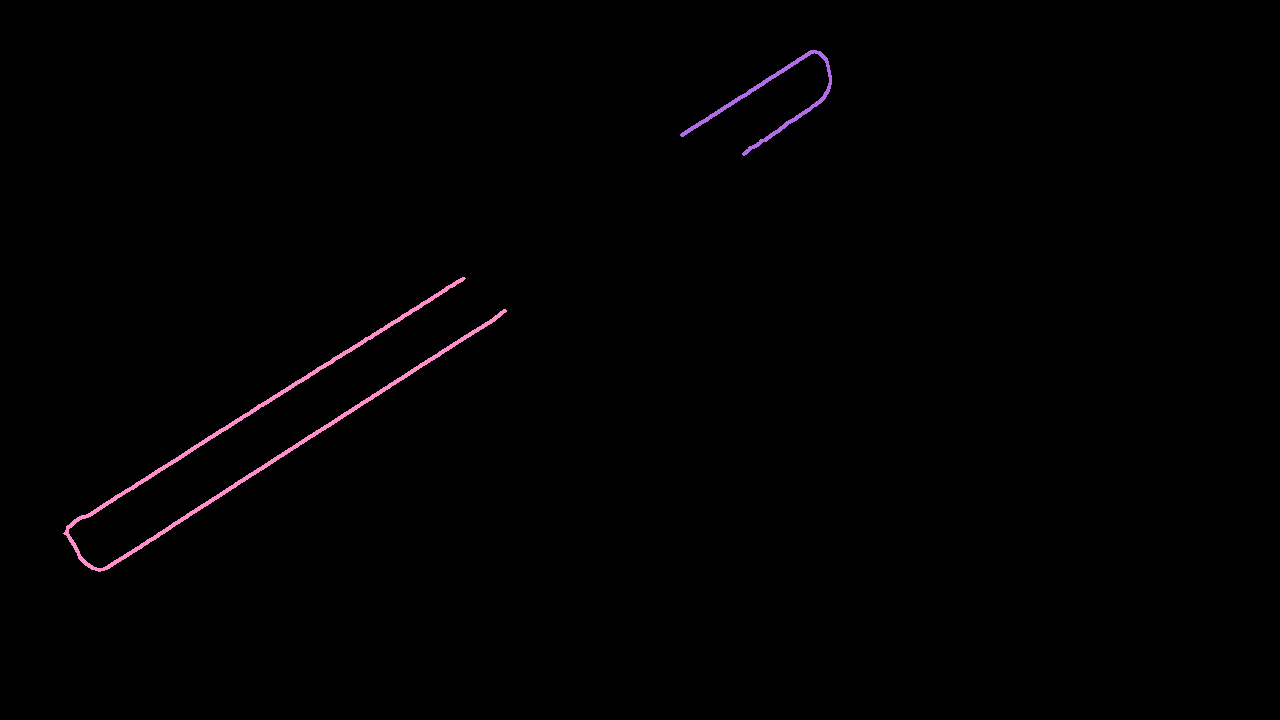 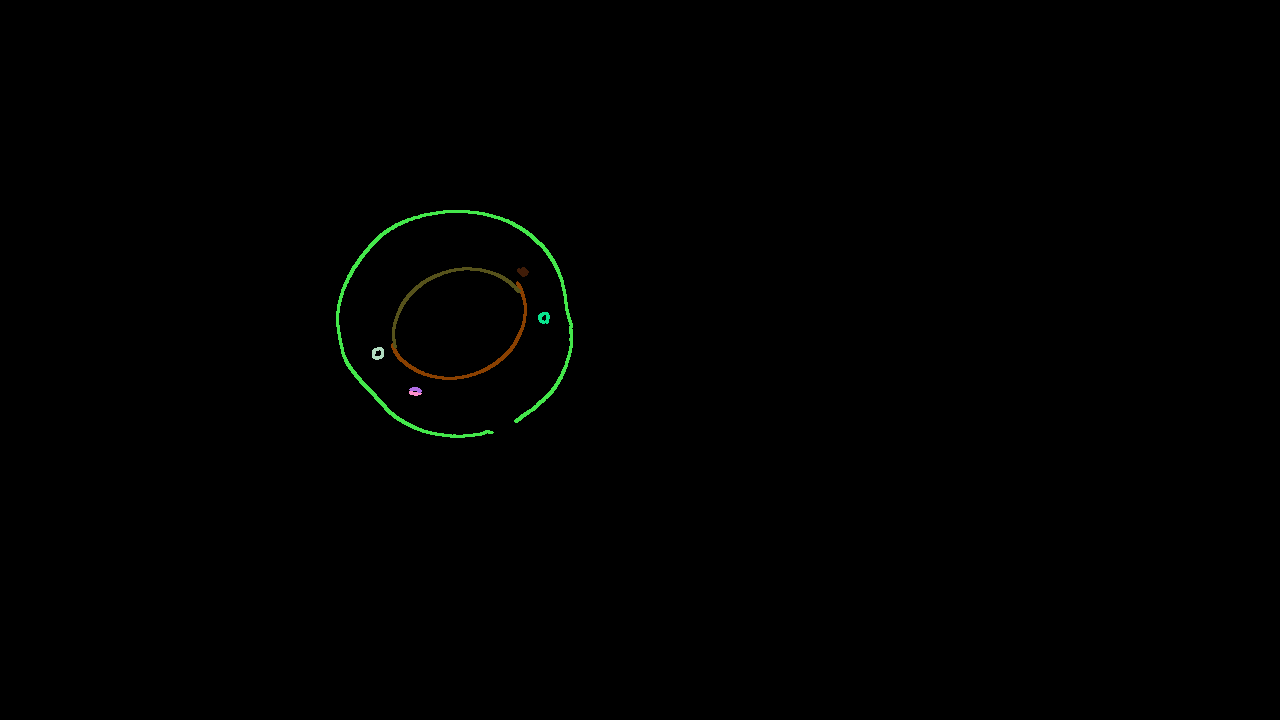 How can I close those contour curves? #include "opencv2/imgproc/imgproc.hpp"
#include "opencv2/highgui/highgui.hpp"
#include <stdlib.h>
#include <stdio.h>
using namespace cv;
RNG rng(12345);
int main()
{
Mat src, srcGray, srcBlur, srcThresh, srcCanny;
src = imread("source.png", 1);
cvtColor(src, srcGray, CV_BGR2GRAY);
blur(srcGray, srcBlur, Size(3, 3));
double otsu;
otsu = threshold(srcBlur, srcThresh, 0, 255, CV_THRESH_BINARY | CV_THRESH_OTSU);
Canny(srcBlur, srcCanny, otsu, otsu * 2, 3, true);
vector<vector<Point> > contours;
vector<Vec4i> hierarchy;
findContours(srcCanny, contours, hierarchy, CV_RETR_EXTERNAL, CV_CHAIN_APPROX_SIMPLE, Point(0, 0));
Mat drawing = Mat::zeros(srcCanny.size(), CV_8UC3);
for (int i = 0; i< contours.size(); i++)
{
Scalar color = Scalar(rng.uniform(0, 255), rng.uniform(0, 255), rng.uniform(0, 255));
drawContours(drawing, contours, i, color, 2, 8, hierarchy, 0, Point());
}
imshow("Contours", drawing);
cvWaitKey();
return 0;
}
I tried something like this but it made it didn't help at all. vector<vector<Point> > approx;
approx.resize(contours.size());
for (intk = 0; k < contours.size(); k++)
approxPolyDP(Mat(contours[k]), approx[k], 3, true);
|
2017-01-15 02:50:03 -0600
| marked best answer | imshow() shows the image after scanf_s() Hello, I would like to get some user input after I show an image. But even though imshow() is being executed, it waits till the user input to show the image. I get an empty window until scanf_s() is done. Why is this happening? I am using Visual Studio 2013, and OpenCV 2.4.11. Here is a little demo: #include <iostream>
#include <opencv2/core/core.hpp>
#include <opencv2/highgui/highgui.hpp>
using namespace cv;
using namespace std;
int main()
{
char in[15];
namedWindow("Source", 1);
Mat src = imread("toy.png");
imshow("Source", src);
scanf_s("%s", in, sizeof in);
waitKey();
return 0;
}
toy.png:
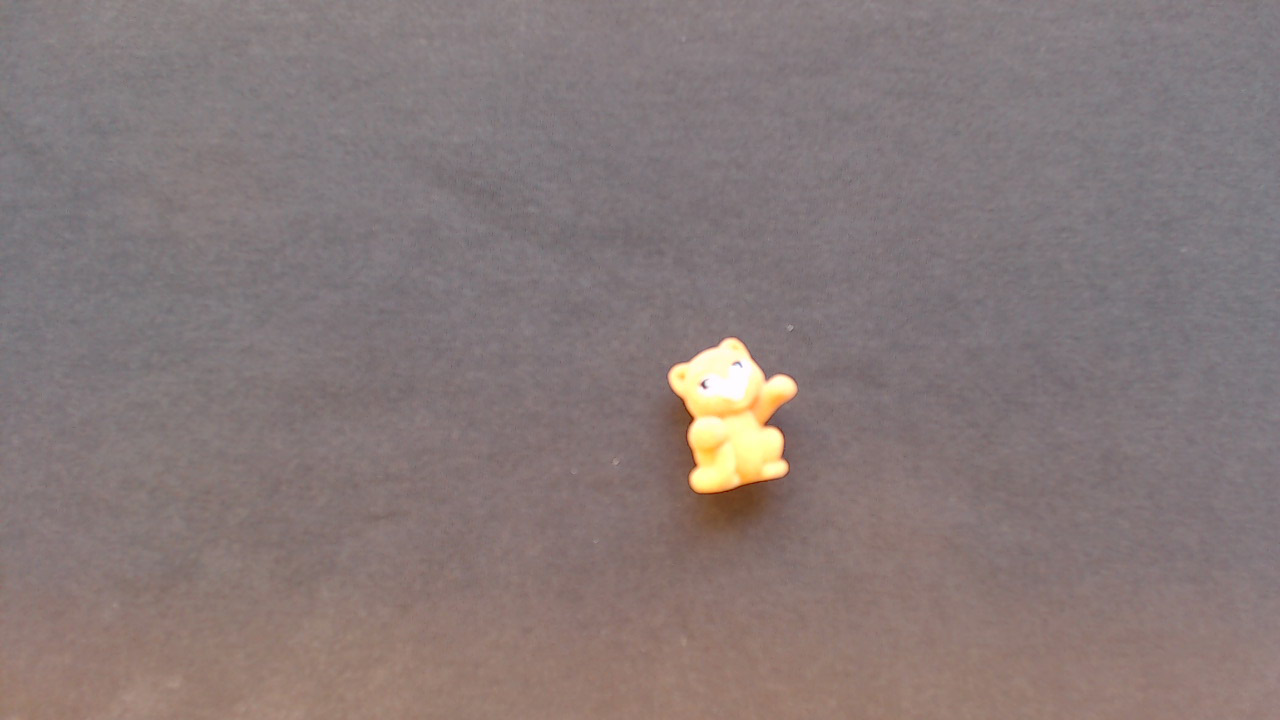 |