In my attempt to implement particle filter, I have first manually drawn a rectangle(x,y,w,h) around the car in my image (in red color), then i took 50 particles, and assigned them noise i.e x=x+noise(0,15) and y=y+noise(0,15).
Then i wanted to draw all the rectangles for each particle in green color, but instead of showing 50 rectangles, it is only showing one rectangle.
#include<opencv2\core\core.hpp>
#include<opencv2\imgproc\imgproc.hpp>
#include<opencv2\highgui\highgui.hpp>
#include<stdio.h>
#include<iostream>
#include<random>
using namespace cv;
using namespace std;
const int N = 50;// no of particles
string intToString(int number){
//this function has a number input and string output
std::stringstream ss;
ss << number;
return ss.str();
}
int main()
{
Mat frame;
frame = imread("f (1).png");
namedWindow("Out");
//locating the car manually
Rect car(175, 210, 42, 31);
//making a rectangle around the car
rectangle(frame, car, Scalar(0, 0,255), 1, 8, 0);
//getting tht height and width of the frame
const int FRAME_HEIGHT = frame.rows;
const int FRAME_WIDTH = frame.cols;
//Particle filter initialization
Mat Init = (Mat_<float>(4, 1) << car.x, car.y, 0, 0);
//for a gaussian noise distribution
std::default_random_engine generator;
std::normal_distribution<double> distribution(0, 15);
//Initializing the particles
std::vector<cv::Mat> particle(N, cv::Mat(4, 1, CV_32F));
cout << car.x << " " << car.y << "\n";
for (int i = 0; i < N; i++)
{
particle[i].at<float>(0, 0) = Init.at<float>(0, 0) + distribution(generator);
particle[i].at<float>(1, 0) = Init.at<float>(1, 0) + distribution(generator);
particle[i].at<float>(2, 0) = 0.0;
particle[i].at<float>(3, 0) = 0.0;
cout << particle[i] << "\n";
}
for (int i = 0; i < N; i++)
{
int x = particle[i].at<float>(0, 0);
int y = particle[i].at<float>(1, 0);
rectangle(frame, Rect(x, y, 42, 31), Scalar(0, 255, 0), 1, 8, 0);
}
imshow("Out", frame);
waitKey();
return 0;
}
The output looks like this
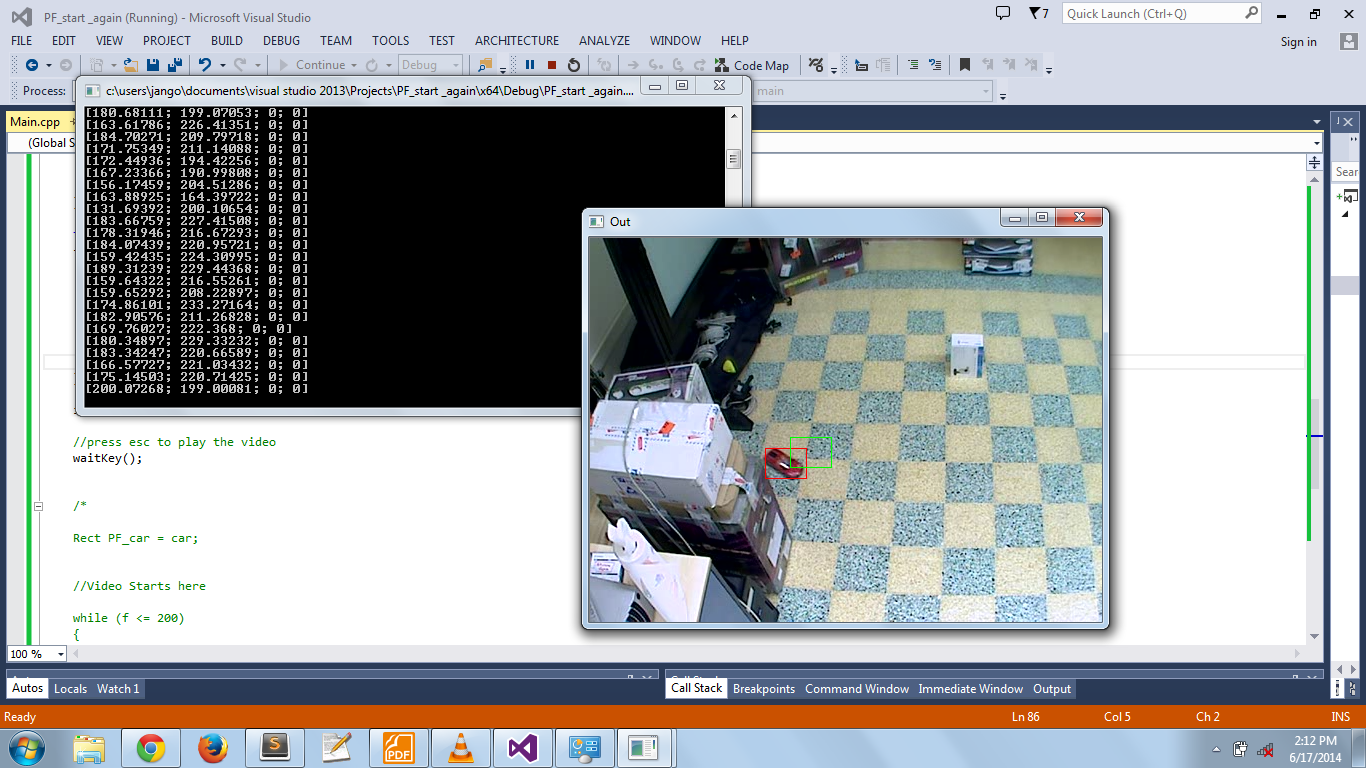
The particle x y coordinates are in the following image, The first entry is of the the car that was manually hard coded, rest all are the particle coordinates.
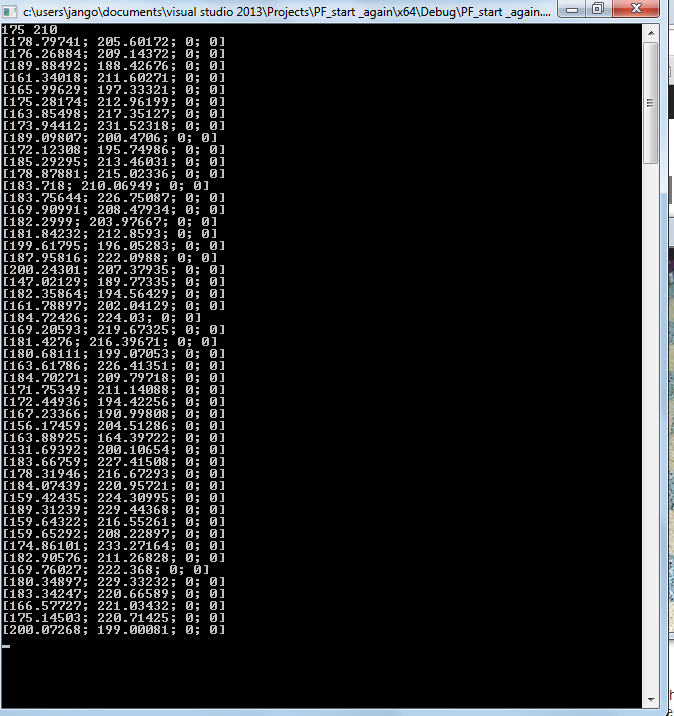