I’m working on a project that should filter the red objects in an image and calculates the distance to this object with two webcams.
To detect the objects i convert the image from BGR to HSV and use the function inRange to threshold them.
Then i use findContours to get the contours in the image, which should be the contours of the red objects.
As last step i use boundingRect to get a Vector of Rect that contains one Rect per detected object.
On the two images below you can see my problem. The one with the pink rectangle is about 162cm away from the camera and the other about 175cm. If the Object is further then 170cm the object is not recognized alltough the thresholded image is showing the contours of the object.
Are the contours of the object just too small or is there any other reason?
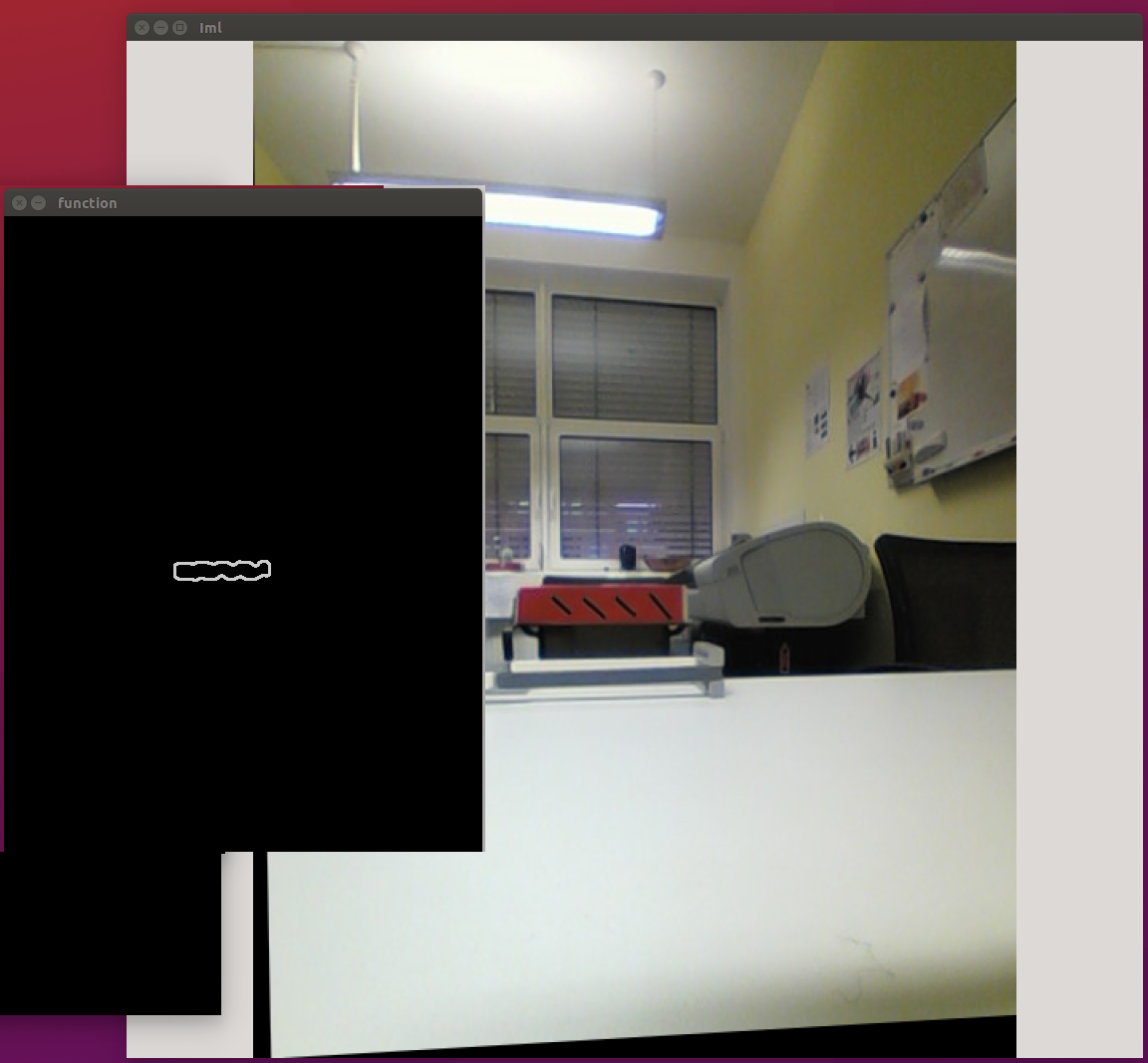
ok heres the code:
main.cpp
ObjectDetection obj;
StereoVision sv;
for (;;) {
cp.read(imgl);
cp2.read(imgr);
sv.calculateDisparity(imgl, imgr, dispfull, disp8, imgToDisplay);
Mat imgrt, imglt;
obj.filterColor(imgl, imgr, imglt, imgrt);
//p1 und p2 sind die gefundenen Konturen eines Bildes
vector<vector<Point> > p1 = obj.getPointOfObject(imglt);
vector<Rect> allRoisOfObjects = obj.getAllRectangles(imgl, p1);
for(int i = 0; i < allRoisOfObjects.size(); i++){
Rect pos = allRoisOfObjects.at(i);
pos.width -= 20;
pos.height -= 20;
pos.x += 10;
pos.y += 10;
disp = dispfull(pos);
float distance = sv.calculateAverageDistance(pos.tl(),pos.br(),dispfull);
stringstream ss;
ss << distance;
rectangle(imgToDisplay, allRoisOfObjects.at(i), color, 2,8, 0);
putText(imgToDisplay, ss.str(), pos.br(), 1, 1, color, 1);
ss.clear();
ss.str("");
newObjects.push_back(pos);
}
}
ObjectDetection.cpp
void ObjectDetection::filterColor(Mat& img1, Mat& img2, Mat& output1,
Mat& output2) {
Mat imgHSV, imgHSV2;
cvtColor(img1, imgHSV, COLOR_BGR2HSV); //Convert the captured frame from BGR to HSV
cvtColor(img2, imgHSV2, COLOR_BGR2HSV);
Mat imgThresholded, imgThresholded2;
inRange(imgHSV, Scalar(iLowH, iLowS, iLowV), Scalar(iHighH, iHighS, iHighV),
imgThresholded);
inRange(imgHSV2, Scalar(iLowH, iLowS, iLowV),
Scalar(iHighH, iHighS, iHighV), imgThresholded2);
output1 = imgThresholded;
output2 = imgThresholded2;
}
vector<vector<Point> > ObjectDetection::getPointOfObject(Mat img) {
vector<vector<Point> > contours;
vector<Vec4i> hierarchy;
findContours(img, contours, hierarchy, CV_RETR_TREE, CV_CHAIN_APPROX_SIMPLE,
Point(0, 0));
return contours;
}
vector<Rect> ObjectDetection::getAllRectangles(Mat & img, vector<vector<Point> > contours){
vector<vector<Point> > contours_poly(contours.size());
vector<Rect> boundRect(contours.size());
vector<Point2f> center(contours.size());
vector<float> radius(contours.size());
Rect rrect;
rrect.height = -1;
RNG rng(12345);
for (int i = 0; i < contours.size(); i++) {
approxPolyDP(Mat(contours[i]), contours_poly[i], 3, true);
boundRect[i] = boundingRect(Mat(contours_poly[i]));
}
return boundRect;
}