I am new in OpenCV and I would like to detect the curvy lines in an image. I tried Hough Transformation, but it detects only the straight line. I want to detect a line something similar to this, but not as curvy as this
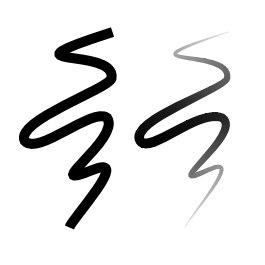
Thank you very much!
Regards
EDIT 1:
import numpy as np
import cv2
# Read the main image
inputImage = cv2.imread("input.png")
# Convert it to grayscale
inputImageGray = cv2.cvtColor(inputImage, cv2.COLOR_BGR2GRAY)
# Line Detection
edges = cv2.Canny(inputImageGray,100,200,apertureSize = 3)
minLineLength = 500
maxLineGap = 5
lines = cv2.HoughLinesP(edges,1,np.pi/180,90,minLineLength,maxLineGap)
for x in range(0, len(lines)):
for x1,y1,x2,y2 in lines[x]:
cv2.line(inputImage,(x1,y1),(x2,y2),(0,128,0),2)
cv2.putText(inputImage,"Tracks Detected", (500,250), font, 0.5, 255)
# Show result
cv2.imshow("Trolley_Problem_Result", inputImage)
This is my code, and the output of this code is (the green line in the image is the result):
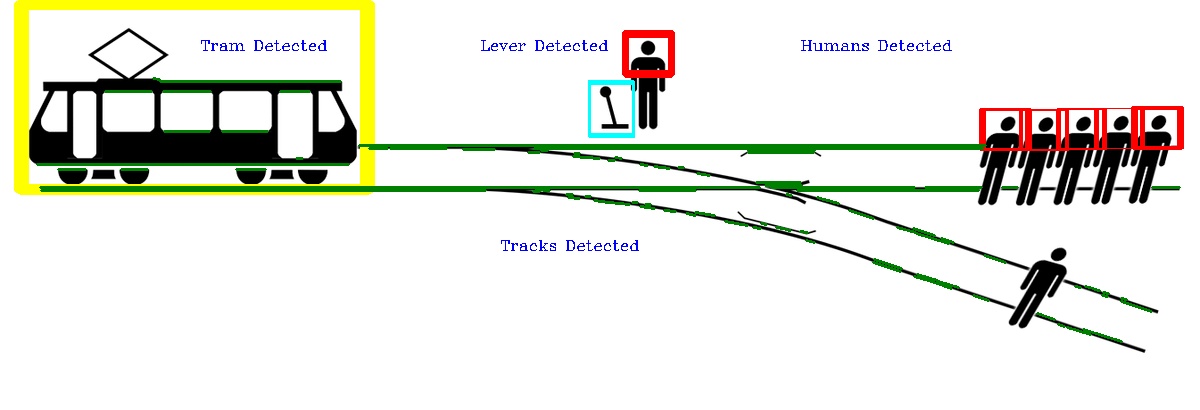
As you can see, the curved tracks are not detected properly. If I increase the threshold value, it detects the lines on the tram (it's detecting now too) and also on the humans.
What do you suggest, so that I can improve the code and detect the curved tracks as well?
Thanks a lot :)
Regards