As already answered by FooBar, yes you can do it.
Just an example code here.
The image to find the templates:
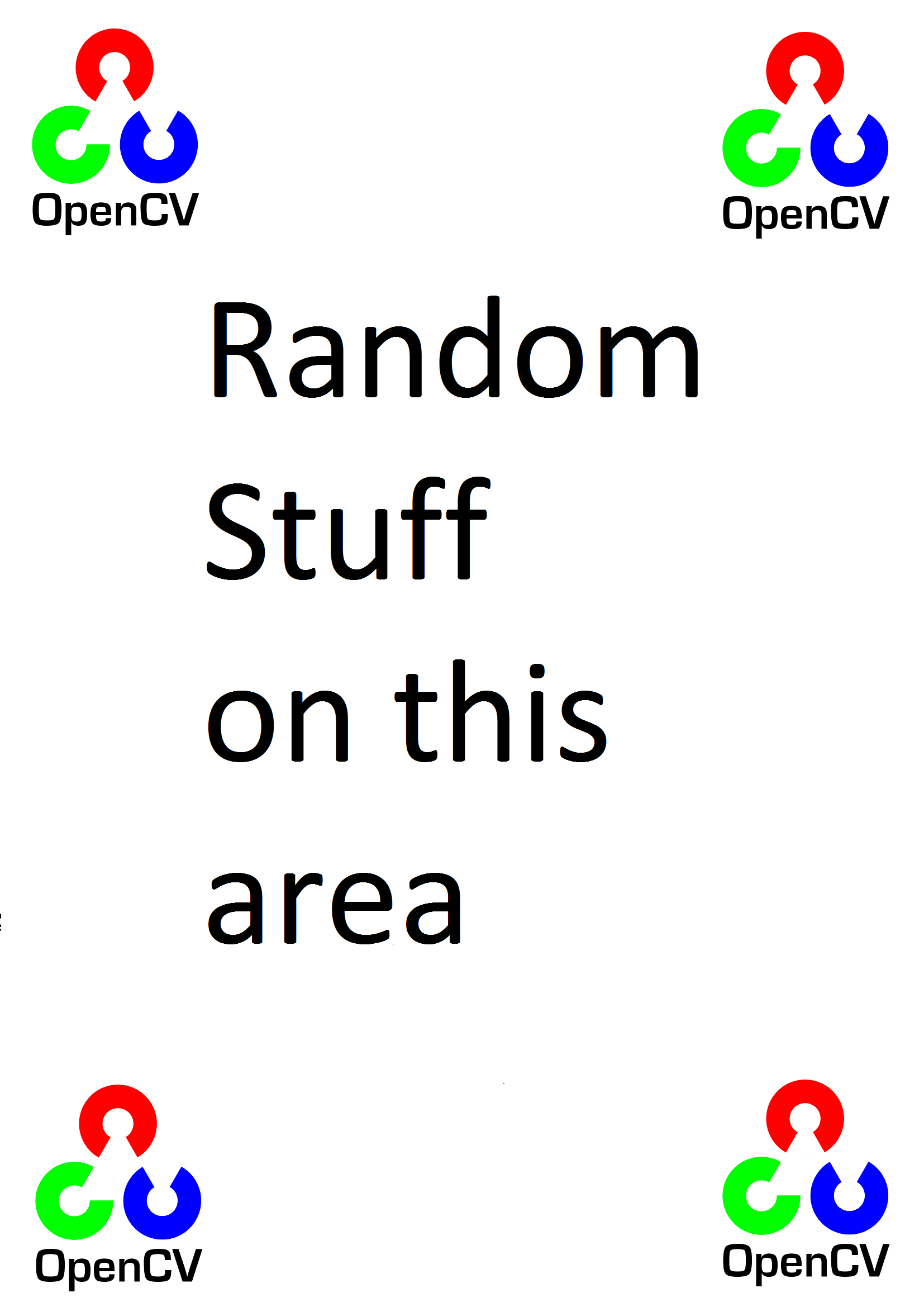
The template image.
#include <iostream>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
//See: http://docs.opencv.org/doc/tutorials/imgproc/histograms/template_matching/template_matching.html
//See: http://answers.opencv.org/question/60382/detect-markers-position-in-2d-images/
int main() {
cv::Mat img, templateImg, result;
cv::VideoCapture capture("http://answers.opencv.org/upfiles/14297307634571599.png");
if(capture.isOpened()) {
capture >> img;
} else {
return -1;
}
capture = cv::VideoCapture("http://answers.opencv.org/upfiles/14297308125543022.png");
if(capture.isOpened()) {
capture >> templateImg;
} else {
return -1;
}
/// Reduce the size of the image to display it on my screen
cv::resize(img, img, cv::Size(), 0.5, 0.5);
/// Reduce the size of the template image
/// (first to fit the size used to create the image test, second to fit the size of the reduced image)
cv::resize(templateImg, templateImg, cv::Size(), 0.25, 0.25);
cv::Mat img_display;
img.copyTo(img_display);
// Create the result matrix
int result_cols = img.cols - templateImg.cols + 1;
int result_rows = img.rows - templateImg.rows + 1;
result.create(result_rows, result_cols, CV_32FC1);
/// Do the Matching and Normalize
cv::matchTemplate(img, templateImg, result, CV_TM_CCORR_NORMED);
cv::normalize(result, result, 0, 1, cv::NORM_MINMAX, -1, cv::Mat());
/// Localizing the best match with minMaxLoc
double minVal; double maxVal; cv::Point minLoc; cv::Point maxLoc;
cv::Point matchLoc;
for(;;) {
cv::minMaxLoc(result, &minVal, &maxVal, &minLoc, &maxLoc, cv::Mat());
matchLoc = maxLoc;
std::cout << "Max correlation=" << maxVal << std::endl;
if(maxVal < 0.8) {
break;
}
/// Show me what you got
cv::rectangle(img_display, matchLoc, cv::Point(matchLoc.x + templateImg.cols , matchLoc.y + templateImg.rows),
cv::Scalar::all(0), 2, 8, 0);
cv::rectangle(result, cv::Point(matchLoc.x - templateImg.cols/2 , matchLoc.y - templateImg.rows/2),
cv::Point(matchLoc.x + templateImg.cols/2 , matchLoc.y + templateImg.rows/2 ), cv::Scalar::all(0), 2, 8, 0);
cv::imshow("result", result);
cv::waitKey(0);
/// Fill the detected location with a rectangle of zero
cv::rectangle(result, cv::Point( matchLoc.x - templateImg.cols/2 , matchLoc.y - templateImg.rows/2),
cv::Point(matchLoc.x + templateImg.cols/2 , matchLoc.y + templateImg.rows/2 ), cv::Scalar::all(0), -1);
} while (maxVal > 0.9);
cv::imshow("result", result);
cv::imshow("img_display", img_display);
cv::waitKey(0);
return 0;
}