You can solve these easily findContours() in your source image and detect whether the contour is unsevered or not by examining the hierarchy passed to the findContours() function. From the first figure it is clearer that no contour has child contour as compared to the second image, you will get this data from hierarchy parameter which is optional output vector, containing information about the image topology. It has as many elements as the number of contours.
Here we will use hierarchy as
vector< Vec4i > hierarchy
where for an i-th contour
- hierarchy[i][0] = next contour at the
same hierarchical level
- hierarchy[i][1] = previous contour at
the same hierarchical level
- hierarchy[i][2] = denotes its first
child contour
- hierarchy[i][3] = denotes index of
its parent contour
If for the contour i there are no next, previous, parent, or nested contours, the corresponding elements of hierarchy[i] will be negative. See findContour() function for more details.
So by checking the value hierarchy[i][2] you can decide the contour belongs to unsevered or not, that is for a contour if the hierarchy[i][2] = -1 then it belongs to unsevered.
And one more thing is that in findContours() function you should use CV_RETR_CCOMP which retrieves all of the contours and organizes them into a two-level hierarchy.
Here is the C++ code how to implement this.
Mat src=imread("src.jpg",1);
cvtColor(src,tmp,CV_BGR2GRAY);
threshold(tmp,thr,200,255,THRESH_BINARY_INV);
vector< vector <Point> > contours; // Vector for storing contour
vector< Vec4i > hierarchy;
findContours( thr, contours, hierarchy,CV_RETR_CCOMP, CV_CHAIN_APPROX_SIMPLE );
for( int i = 0; i< contours.size(); i=hierarchy[i][0] ) // iterate through each contour.
{
Rect r= boundingRect(contours[i]);
if(hierarchy[i][2]<0) //Check if there is a child contour
rectangle(src,Point(r.x,r.y), Point(r.x+r.width,r.y+r.height), Scalar(0,255,0),2,8,0);
else
rectangle(src,Point(r.x,r.y), Point(r.x+r.width,r.y+r.height), Scalar(0,0,255),2,8,0);
}
imshow("src",src);
waitKey();
Result: Here the green rectangle represent the positive object and red represent the false object.
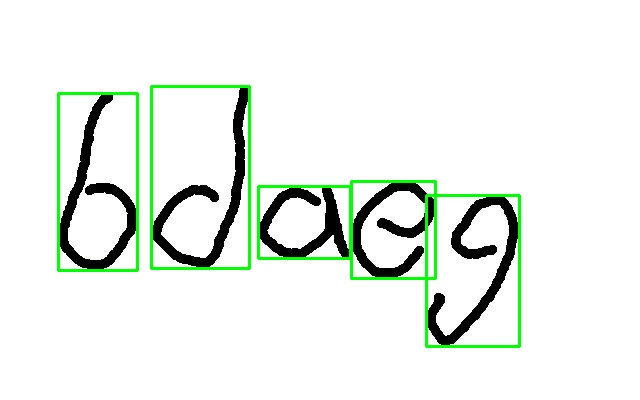
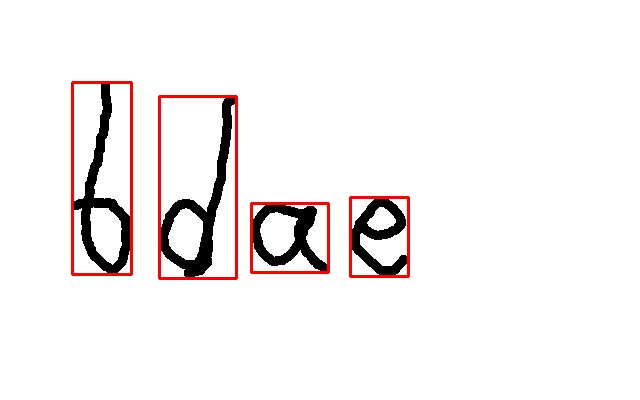