Hello Santhosh!
Here is a Sample Implementation to find the Edge points of a Contour! Hope this helps!
#include <opencv2\opencv.hpp>
#include "opencv2\opencv_modules.hpp"
using namespace cv;
using namespace std;
int _tmain(int argc, _TCHAR* argv[])
{
Mat mSrc= imread("D:\\Conti\\Image Samples\\forum\\Test.png",0);
if(mSrc.empty())
{
cout<< "Invalid Input Image!";
return 0;
}
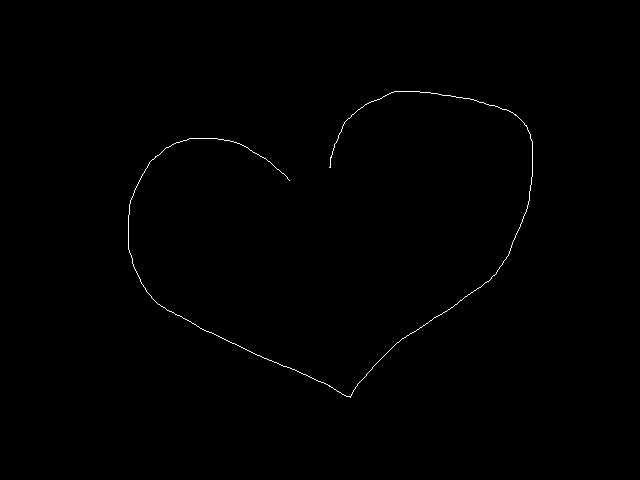
Mat mSrc_Binary,mSrc_Contours,mEndPoints,mResult;
//Creating a Binary Image
threshold(mSrc,mSrc_Binary,1,255,THRESH_BINARY);
//Make sure it is one Pixel width .i.e to replicate a contour Points
thinning(mSrc_Binary,mSrc_Contours);
Mat_<int> Kernal(3,3);
Kernal << 1, 1, 1,
1,10, 1,
1, 1, 1;
//Multiply the Binary Image with the above Kernal
//Credits & Original Author:
https://stackoverflow.com/questions/26537313/how-can-i-find-endpoints-of-binary-skeleton-image-in-opencv
/*
0 0 1 0 * Kernal 1, 1, 1 => 1 3 12 2
0 1 0 0 1,10, 1 1 11 2 1
0 0 0 0 1, 1, 1 1 1 1 0
*/
filter2D(mSrc_Contours,mEndPoints,CV_8UC1,Kernal);
//We are interested in the pixels whicn are equal to 11
inRange(mEndPoints,11,11,mEndPoints);
//Just adding some Visualization!!
cvtColor(mSrc_Binary,mResult,COLOR_GRAY2BGR);
dilate(mEndPoints, mEndPoints, Mat(), Point(-1, -1), 2, 1, 1);//Make it Visible
mResult.setTo(Scalar(0,0,255),mEndPoints);
imshow("mResult",mResult);
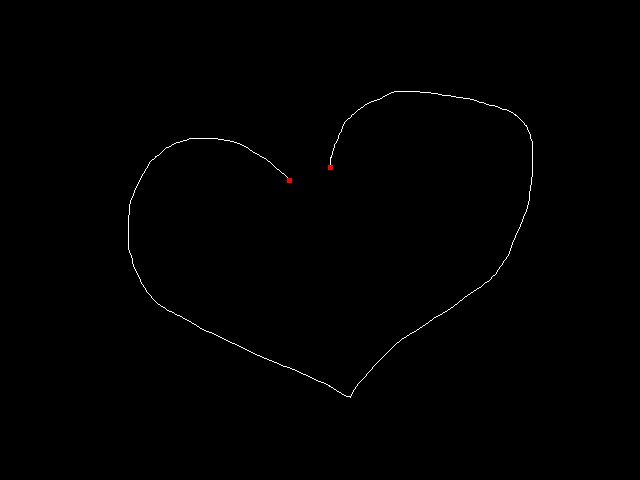
waitKey();
return 0;
}
dilate and use thinning. It should be available in python)
@LBerger I don't think dilate or thinning is required here. I already have my discrete points(x,y) which I'm interested in. I just need the two end points.
If you know the answer to your question why do you ask a question? I see on your image that end points got only one neighbour for a distance less than a threshold. If you have another definition post it
You can use voronoi, dilate and thinning or flann
@LBerger I have just selected every 6 consecutive point
select[::6]
a particular detected contour and plotted them here. The contour detected is not in order from one end to the other end point. It could have been detected from the middle of the contour or any other part of the contour and end at any other point.Ok so try to remove all double points in your array (double point= cross twice in your list)
@LBerger I have already removed the duplicate points by using
np.unique
beforeselect[::6]
. Could you please explain what this double point logic is?When you iterate through your array add 1 to an empty image. Some points will be iterate twice : remove this points: end points only one. If thickness line is not one some points will be iterate only one time : use thinin algorithm (you must fill your shape first)
@LBerger Points iterated twice have already been remove by using
np.unique
I still am confused.