You can use binarization with a threshold on the brightness (L-channel of HSL color space). Then you can apply binarization and opening / closing.
Python sample code
#!/usr/bin/env python
from PIL import Image
import numpy as np
from scipy.misc import imsave
from scipy.ndimage.morphology import binary_closing, binary_opening
def binarize_array(numpy_array, threshold=200):
"""
Binarize a numpy array.
Source: https://stackoverflow.com/a/37497975/562769
Parameters
----------
numpy_array : numpy array
threshold : int
Returns
-------
numpy_array
"""
for i in range(len(numpy_array)):
for j in range(len(numpy_array[0])):
if numpy_array[i][j] > threshold:
numpy_array[i][j] = 255
else:
numpy_array[i][j] = 0
return numpy_array
im = Image.open("14810202126365282.jpg")
im = im.convert('L')
im = np.array(im)
im = binarize_array(im, 200)
im = binary_closing(im, structure=np.ones((10, 10)))
im = binary_opening(im, structure=np.ones((10, 10)))
imsave("out.png", im)
Sample mask for the book
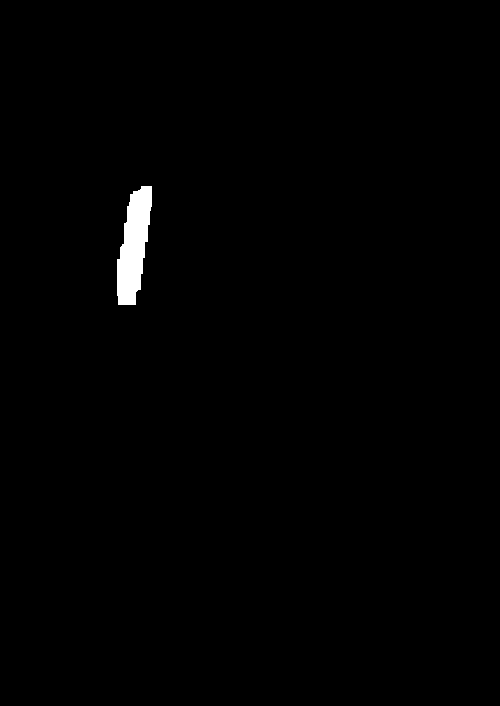
have you read http://answers.opencv.org/question/92... and this paper http://yima.csl.illinois.edu/psfile/r... (with code on website)
LBerger, this method didn't work with images where no specularities. When I try this method for first object (book) which image has not specularities, it detect speculars on label (white letters).
Your link to paper seems to be good, I still read it, thank you!