I'm trying to reproduce the same output with these snippets:
Scikit-Image + Keras
from keras.models import model_from_json
import numpy as np
from skimage.io import imread
from skimage.transform import resize
image = resize(imread(img_path, as_grey=False), (80, 80), preserve_range=True, mode='constant')
image /= 255.
img_array = np.array([image])
pred_IN = model.predict(img_array)
OpenCV
import cv2
model = cv2.dnn.readNet('mynet.prototxt', 'mynet.caffemodel')
image = cv2.imread(image_path)
img = cv2.dnn.blobFromImage(image, scalefactor=(1.0/255.0), size=(80, 80), swapRB=True, crop=False)
model.setInput(img)
pred = model.forward()
The problem is that I cannot get the same data to pass to the network (DNN module in case of OpenCV). Network is the same, input data is the same, but the results is slightly different and the reason is that resize function behaves differently between scikit-image and OpenCV (used internally by blobFromImage) and don't know how to adapt the OpenCV code to match scikit-image. scikit-image.
My final application will use OpenCV in C++, so I need to match this snippets, as my network has been trained with data generated by scikit-image.
EDIT
I think the problem is that Resize function of OpenCV (used internally by blobFromImage) produce a different result from scikit-image resize (I'm not saying is bugged, just want to obtain same results bewteen OpenCV and Sciki-Image, doing something in one or in another), for example for this image:
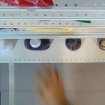
image32 = cv2.imread(image).astype(np.float32)
cv2.cvtColor(image32,cv2.COLOR_BGR2RGB,image32)
new_image = cv2.resize(image32,(80,80),interpolation=cv2.INTER_LINEAR)
Upper left pixel value is: 145.91113, 76.853516, 92.67676
But with scikit-image:
img = resize(imread(img_path, as_grey=False), (80, 80), preserve_range=True, mode='constant')
Upper left pixel value is: 144.09179688, 75.74609375, 9.85742188
Scikit image uses by default interpolation bi-linear, the same used by OpenCV, so it should be the same, I also tried every possible value for parameter mode, I can never obtain the same result between scikit and OpenCV.
Altough the values seems only slightly different, when passed to the network, the network produce a different result, which I noticed can be up to 12% in classification probalility. (In this case is only 3%, top class has 0.7 with scikit + Keras and 0.66 with OpenCV).