After I masked the image and get the part I wanted. The next process is to count the black and white pixels of the unmasked part of the image but I don't know how to do it. Anyone who can give me the method to solve this.
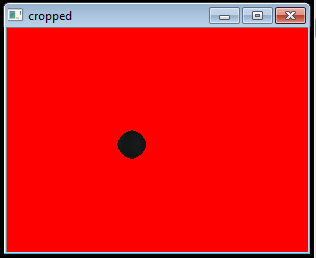
#include "opencv\cvaux.h"
#include "opencv\cxmisc.h"
#include "opencv\highgui.h"
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <stdlib.h>
using namespace std;
using namespace cv;
int main(int, char**)
{
Mat threshold_output;
vector<vector<point> > contours;
vector<vec4i> hierarchy;
RNG rng(12345);
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/highgui/highgui.hpp>
using namespace cv;
int main(int argc, char** argv)
CvCapture* capture = cvCaptureFromCAM(0);
cvSetCaptureProperty(capture, CV_CAP_PROP_FRAME_WIDTH, 270);
cvSetCaptureProperty(capture, CV_CAP_PROP_FRAME_HEIGHT, 190);
cv::Mat frame; cv::Mat src_gray;
while(true) {
Mat src frame = imread("D:/sapsaphead3.jpg");
Mat gray;
cvtColor(~src, gray, CV_BGR2GRAY);
medianBlur(gray,gray,27);
threshold(gray, gray, 220, 255, THRESH_BINARY);
vector<vector<Point> > contours;
findContours(gray.clone(), contours, CV_RETR_EXTERNAL, CV_CHAIN_APPROX_NONE);
cvQueryFrame( capture );
cvtColor( frame,src_gray, CV_BGR2GRAY );
medianBlur( src_gray, src_gray, 25 );
threshold( src_gray.clone(), threshold_output, 20, 255,CV_THRESH_BINARY_INV);
findContours( threshold_output.clone(), contours,hierarchy,CV_RETR_EXTERNAL, CV_CHAIN_APPROX_SIMPLE, Point(0, 0) );
for (int i = 0; i < contours.size(); i++)
{
double area =contourArea(contours[i]);
Rect rect = boundingRect(contours[i]);
if (area >= 50 30 && abs(1 - ((double)rect.width / (double)rect.height)) /(double)rect.height)) <= 0.2)
0.1)
{
Mat mask = Mat::zeros(gray.rows, gray.cols, Mat::zeros(threshold_output.rows, threshold_output.cols, CV_8UC1);
drawContours( mask, contours, i, CV_RGB(255,255,255), -1, 8, vector<Vec4i>(), 0,
Point() );
contours,i , Scalar::all(255), -1);
Mat crop(src.rows, src.cols, crop(frame.rows, frame.cols, CV_8UC3);
crop.setTo(Scalar(255,255,255));
src.copyTo(crop, frame.copyTo(crop, mask);
int count_black = 0;
int count_white = 0;
int black_total;
int white_total;
for( int y = 0; y < src.rows; frame.rows; y++ )
{
for( int x = 0; x < src.cols; frame.cols; x++ )
{
if ( mask.at<uchar>(y,x) != 0 ) {
// change this to to 'src.atuchar>(y,x) == 255'
// if your img has only 1 channel
if ( src.at<cv::Vec3b>(y,x) == cv::Vec3b(255,255,255) )
{
count_white++;
}
else if ( src.at<cv::Vec3b>(y,x) frame.at<cv::Vec3b>(y,x) == cv::Vec3b(0,0,0) )
{
count_black++;
black_total = count_black + count_black ;
printf("black= %d",black_total);
}
}
printf("count_white = %d\n",count_white);
printf("count_black = %d\n",count_black);
}
}
imshow( "frame", frame );
imshow("crop",crop);
waitKey(33);
}
}
imshow("cropped", crop);
waitKey(0);
}
}
}