Need some help, i am not able to find the desired contour
Input: 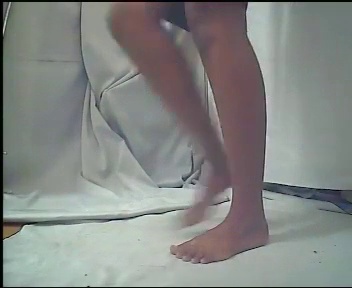
After applying binary filter: 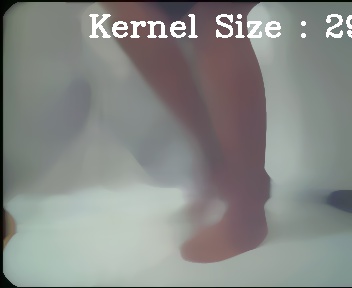
After applying HSV filter:
HSV threshold code:
include "opencv2/imgproc/imgproc.hpp"
include "opencv2/highgui/highgui.hpp"
include "opencv2/video/video.hpp"
include "opencv2/objdetect/objdetect.hpp"
include <opencv2 opencv.hpp="">
include <iostream>
include <opencv2 ml.hpp="">
using namespace std;
using namespace cv;
int main()
{
Size frameSize(500, 400);
Mat frame = imread("C:/Proj/Dissertation/HSV/HSVimage.jpg", imread("C:/Proj/HSVimage.jpg", CV_LOAD_IMAGE_COLOR);
Size srcSize(frame.size());
Mat hsv(srcSize, 3);
vector<mat> hsvMask;
Mat hImg(srcSize, 1);
Mat sImg(srcSize, 1);
cvtColor(frame, hsv, CV_BGR2HSV);
//Vec3b hsv = hsv.at<vec3b>(0, 0);
split(hsv, hsvMask);
hImg = hsvMask[0];
sImg = hsvMask[1];
imshow("hImg", hImg);
int h;
for (int i = 0; i < hImg.rows; i++)
{
for (int a = 0; a < hImg.cols; a++)
{
h = hImg.at<uchar>(i, a);
if (h >= 0 && h <= 25)
{
hImg.at<uchar>(i, a) = 255;
}
else
{
hImg.at<uchar>(i, a) = 0;
}
}
}
imshow("HSV Image", hImg);
imwrite("HSVThreshold.jpg",hImg);
waitKey(0);
return 0;
}
After thresholding the HSV image: 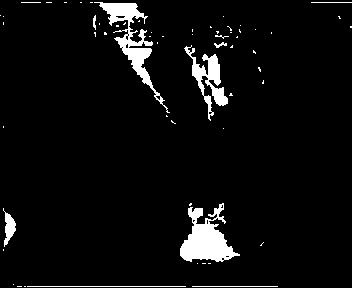
Output: 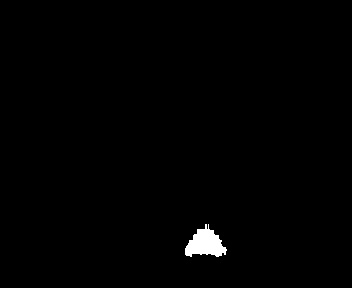
However, the desired output should have been: 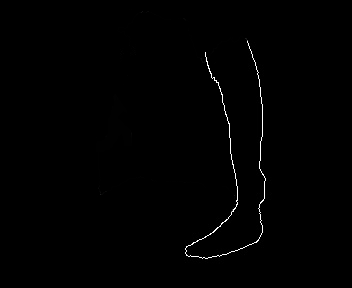
Need some help, this is my code for hsv:
include "opencv2/core/core.hpp"
include "opencv2/highgui/highgui.hpp"
include "opencv2/imgproc/imgproc.hpp"
include "iostream"
using namespace cv;
using namespace std;
int main()
{
Mat image;
image = imread("C:/Proj/Denoised.jpg", CV_LOAD_IMAGE_COLOR);
if (!image.data)
{
cout << "Could not open the denoised image" << std::endl;
return -1;
}
// Create a new matrix to hold the hsv image
Mat HSV;
// convert RGB image to hsv image
cvtColor(image, HSV, CV_BGR2HSV);
namedWindow("Display RGB image", CV_WINDOW_AUTOSIZE);
imshow("Display RGB image", image);
//DISPLAY image
namedWindow("Result : HSV image", CV_WINDOW_AUTOSIZE);
imshow("Result : HSV image", HSV);
//save the HSV image
imwrite("C:/Proj/HSVimage.jpg", HSV);
waitKey(0);
return 0;
}