![]() | 1 | initial version |
Hi @beny2111! If you want to compare multiple Template Images in a Scene Image you have to follow the following steps.
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include <iostream>
#include <stdio.h>
using namespace std;
using namespace cv;
bool FindTemplate(Mat Img_Scene_Bgr,Mat Img_Template_Bgr,Point &Point_TemplateLocation)
{
// `Img_Scene_Bgr` and `Img_Template_Bgr` are the reference and template image
cv::Mat Img_Result_Float(Img_Scene_Bgr.rows-Img_Template_Bgr.rows+1, Img_Scene_Bgr.cols-Img_Template_Bgr.cols+1, CV_32FC1);
cv::matchTemplate(Img_Template_Bgr, Img_Scene_Bgr, Img_Result_Float, CV_TM_CCOEFF_NORMED);
normalize( Img_Result_Float, Img_Result_Float, 0, 1, NORM_MINMAX, -1, Mat() );
double minval, maxval, threshold = 0.7;
cv::Point minloc, maxloc;
cv::minMaxLoc(Img_Result_Float, &minval, &maxval, &minloc, &maxloc);
if (maxval >= threshold)
{
Point_TemplateLocation= maxloc;
return true;
}
else
{
return false;
}
}
int main( int argc, char** argv )
{
Mat Img_Scene;
Mat Img_Template_1;
Mat Img_Template_2;
Mat Img_Result;
char* image_window = "Source Image";
char* result_window = "Result window";
/// Load image and template
Img_Scene = imread("SceneImage.png", 1 );
Img_Template_1 = imread( "Templ1.png", 1 );
Img_Template_2 = imread( "Templ2.png", 1 );
if(Img_Scene.data== NULL||Img_Template_1.data==NULL||Img_Template_2.data==NULL)
{
cout<<"Image Not Found";
return 0;
}
Img_Result= Img_Scene.clone();
Vector<Mat> List_Template_Img;
List_Template_Img.push_back(Img_Template_1);//Otherwise Get some folder & add the Files in it
List_Template_Img.push_back(Img_Template_2);
Point Point_TemplateLocation;
for (int i = 0; i < List_Template_Img.size(); i++)
{
if(!FindTemplate(Img_Scene,List_Template_Img[i],Point_TemplateLocation))
{
cout<<"No Match Found";
}
/// Show me what you got
rectangle( Img_Result, Point_TemplateLocation, Point( Point_TemplateLocation.x + Img_Template_1.cols , Point_TemplateLocation.y + Img_Template_1.rows ), Scalar(0,0,255), 2, 8, 0 );
putText( Img_Result, format("Object %d ",i),Point( Point_TemplateLocation.x + Img_Template_1.cols/4 , Point_TemplateLocation.y + Img_Template_1.rows/2 ),1,1,Scalar(255,0,0),1,-1);
}
/// Create windows
namedWindow( image_window, CV_WINDOW_AUTOSIZE );
namedWindow( result_window, CV_WINDOW_AUTOSIZE );
imshow( image_window, Img_Template_1);
imshow( image_window, Img_Template_2);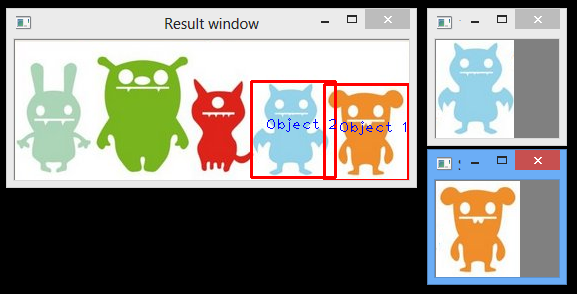
imshow( result_window, Img_Result );
waitKey(0);
return 0;
}
![]() | 2 | edited allignment |
Hi @beny2111! If you want to compare multiple Template Images in a Scene Image you have to follow the following steps.
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include <iostream>
#include <stdio.h>
using namespace std;
using namespace cv;
bool FindTemplate(Mat Img_Scene_Bgr,Mat Img_Template_Bgr,Point &Point_TemplateLocation)
{
// `Img_Scene_Bgr` and `Img_Template_Bgr` are the reference and template image
cv::Mat Img_Result_Float(Img_Scene_Bgr.rows-Img_Template_Bgr.rows+1, Img_Scene_Bgr.cols-Img_Template_Bgr.cols+1, CV_32FC1);
cv::matchTemplate(Img_Template_Bgr, Img_Scene_Bgr, Img_Result_Float, CV_TM_CCOEFF_NORMED);
normalize( Img_Result_Float, Img_Result_Float, 0, 1, NORM_MINMAX, -1, Mat() );
double minval, maxval, threshold = 0.7;
cv::Point minloc, maxloc;
cv::minMaxLoc(Img_Result_Float, &minval, &maxval, &minloc, &maxloc);
if (maxval >= threshold)
{
Point_TemplateLocation= maxloc;
return true;
}
else
{
return false;
}
}
int main( int argc, char** argv )
{
Mat Img_Scene;
Mat Img_Template_1;
Mat Img_Template_2;
Mat Img_Result;
char* image_window = "Source Image";
char* result_window = "Result window";
/// Load image and template
Img_Scene = imread("SceneImage.png", 1 );
Img_Template_1 = imread( "Templ1.png", 1 );
Img_Template_2 = imread( "Templ2.png", 1 );
if(Img_Scene.data== NULL||Img_Template_1.data==NULL||Img_Template_2.data==NULL)
{
cout<<"Image Not Found";
return 0;
}
Img_Result= Img_Scene.clone();
Vector<Mat> List_Template_Img;
List_Template_Img.push_back(Img_Template_1);//Otherwise Get some folder & add the Files in it
List_Template_Img.push_back(Img_Template_2);
Point Point_TemplateLocation;
for (int i = 0; i < List_Template_Img.size(); i++)
{
if(!FindTemplate(Img_Scene,List_Template_Img[i],Point_TemplateLocation))
{
cout<<"No Match Found";
}
/// Show me what you got
rectangle( Img_Result, Point_TemplateLocation, Point( Point_TemplateLocation.x + Img_Template_1.cols , Point_TemplateLocation.y + Img_Template_1.rows ), Scalar(0,0,255), 2, 8, 0 );
putText( Img_Result, format("Object %d ",i),Point( Point_TemplateLocation.x + Img_Template_1.cols/4 , Point_TemplateLocation.y + Img_Template_1.rows/2 ),1,1,Scalar(255,0,0),1,-1);
}
/// Create windows
namedWindow( image_window, CV_WINDOW_AUTOSIZE );
namedWindow( result_window, CV_WINDOW_AUTOSIZE );
imshow( image_window, Img_Template_1);
imshow( image_window, Img_Template_2);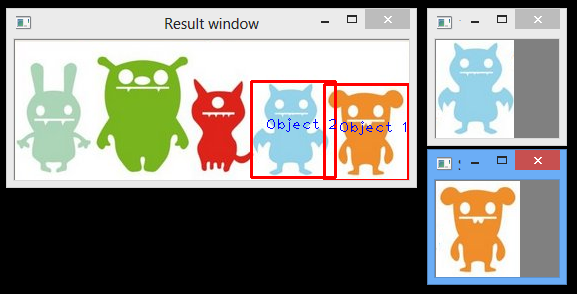
Img_Template_2);
imshow( result_window, Img_Result );
waitKey(0);
return 0;
}