- OpenCV version: 3.1
- Host OS: Mac OS X 10.11.4
Issue in imgproc -> Floodfill function
I have a method that uses floodfill function on an image. The issue is that I get different results when running the code on different machines, even with same opencv version.
Has anyone else experienced this issue?
I can't figure out what is causing this.
original image:
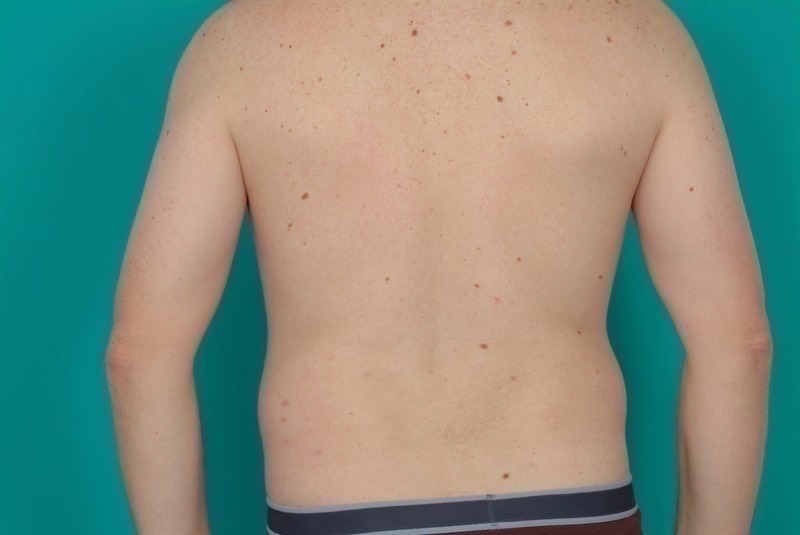
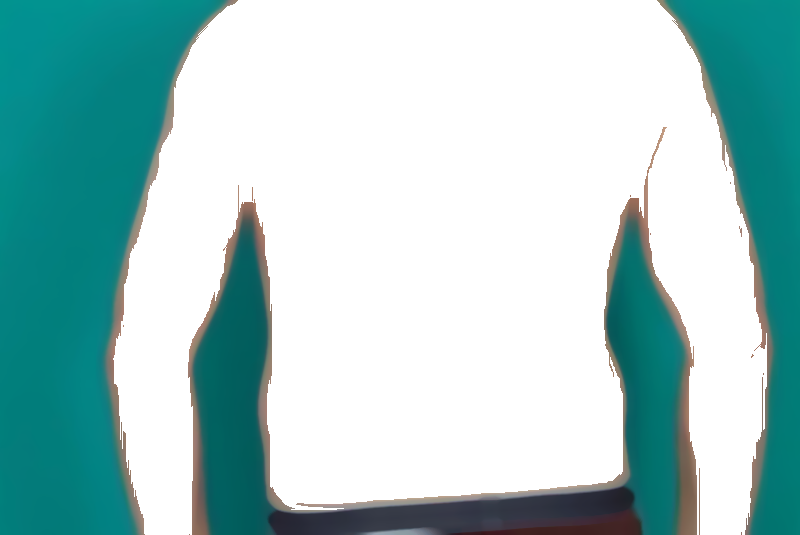
```
include <iostream>
include <string>
include <time.h>
include <ctime>
include <cmath>
include <opencv2 core="" core.hpp="">
include <opencv2 imgproc="" imgproc.hpp="">
include <opencv2 highgui="" highgui.hpp="">
using namespace std;
Mac OS X EI Capitan, OpenCV version: 3.1:
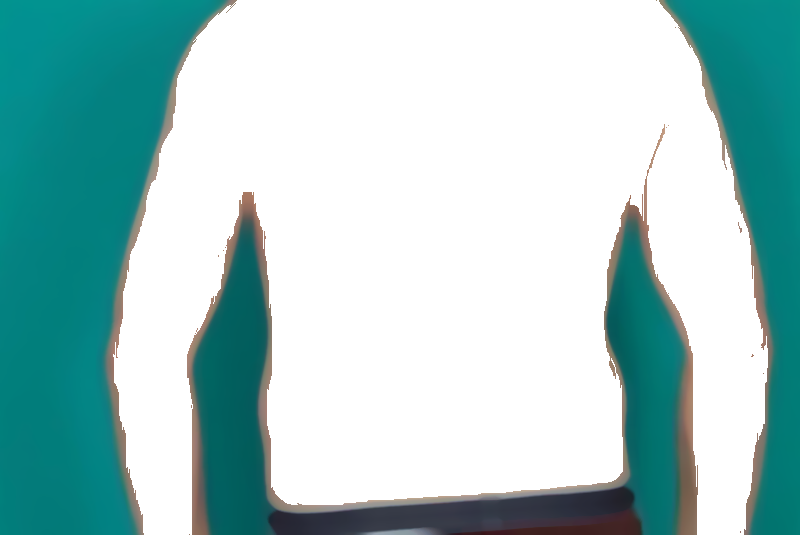
Ubuntu 14.04.4 LTS, OpenCV version: 3.1:
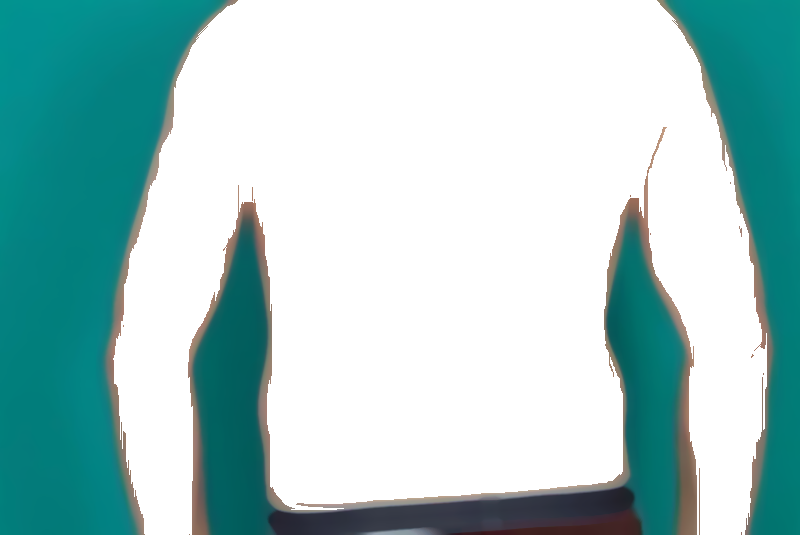
`
void findMaxHistogram(cv::Mat input, cv::Mat mask, int& maxIdx)
{
cv::Mat hist; int histSize = 256;
float range[] = { 0, 256 } ;
const float* histRange = { range };
calcHist( &input, 1, 0, mask, hist, 1, &histSize, &histRange, true, false );
cv::minMaxIdx(hist, NULL, NULL, NULL , &maxIdx);
}
int main(int argc, const char * argv[]) {
string fileName_Ref = //path to original image
//original_image_path
cv::Mat refImage = cv::imread(fileName_Ref);
cv::Mat blurredImage;
cv::medianBlur(refImage, blurredImage,19);
cv::imshow("blurred_image", blurredImage);
cv::imwrite("compare_blur.png", blurredImage);
cv::Mat gray_img;
cv::cvtColor(blurredImage, gray_img, cv::COLOR_BGR2GRAY);
cv::Rect rect = cv::Rect(refImage.cols/2, refImage.rows/5, 1, 3*refImage.rows/5);
cv::Mat mid_col = gray_img(rect);
int maxIdx;
findMaxHistogram(mid_col, cv::Mat(), maxIdx);
cout << maxIdx << '\n';
for( int y = refImage.rows/5; y <= 4 *blurredImage.rows/5; y++ )
{
if (gray_img.at<uchar>(y,blurredImage.cols/2) == maxIdx)
{
floodFill( blurredImage, cv::Mat(), cv::Point(blurredImage.cols/2,y), cv::Vec3b(255,255,255), 0, cv::Scalar::all(1), cv::Scalar::all(1) , 8 | 255<<8 );
break;
}
}
cv::imshow("ff_blurred_image", cv::imshow("floodfill_result", blurredImage);
cv::imwrite("compare_ff_blur.png", blurredImage);
cv::waitKey();
return 0;
}
````