Hi,
I just downloaded OpenCV today. I decided to give it a test on a really simple example.
So I did Hough Circles on this simple image:
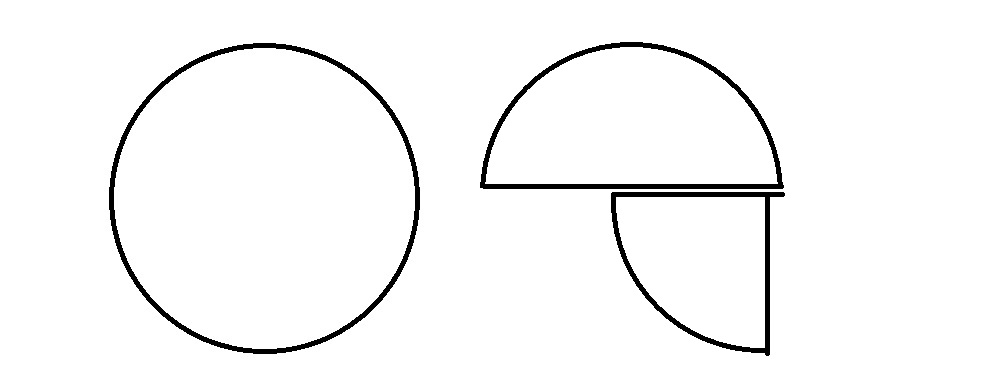
And I implemented Hough Circle using python :
import cv2
import numpy as np
img = cv2.imread('a_circle.jpg',0)
gray = cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
cimg = cv2.cvtColor(img,cv2.COLOR_GRAY2BGR)
bin=cv2.threshold(img,0,255,cv2.THRESH_BINARY+cv2.THRESH_OTSU)[1]
invbin=cv2.bitwise_not(bin)
edges = cv2.Canny(img,50,150,apertureSize = 3)
circles = cv2.HoughCircles(edges,cv2.HOUGH_GRADIENT,1,20,param1=50,param2=30,minRadius=100,maxRadius=0)
cv2.imshow('edge',edges)
cv2.waitKey(0)
circles = np.uint16(np.around(circles))
for i in circles[0,:]:
# draw the outer circle
cv2.circle(cimg,(i[0],i[1]),i[2],(0,255,0),2)
# draw the center of the circle
cv2.circle(cimg,(i[0],i[1]),2,(0,0,255),3)
cv2.imshow('detected circles',cimg)
cv2.waitKey(0)
cv2.destroyAllWindows()
However, I got a very messy circle detection with too many circles!
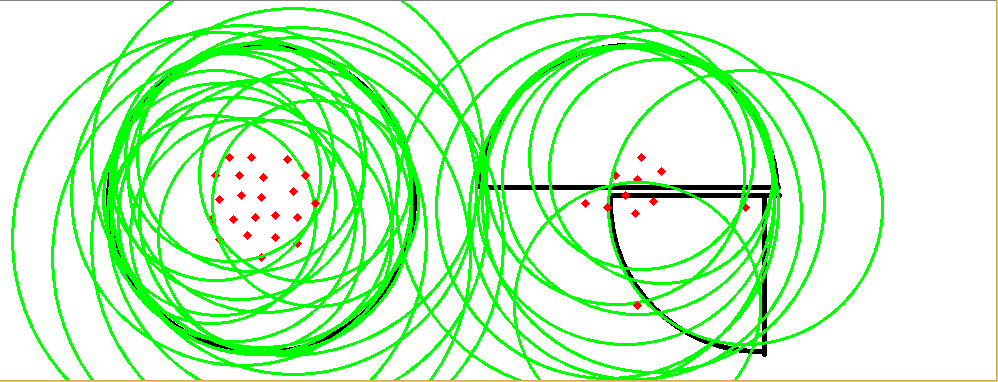
I must be doing something wrongly! Could you tell me what it is?
Thanks very much!
Update:
I changed minDistance from 20 to 50 and I got:
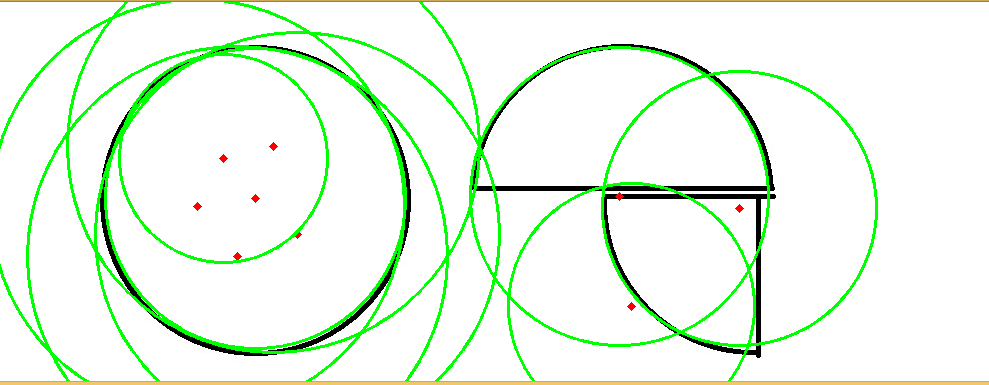
Update 2:
I changed minDistance from 50 to 120 and I got:
The problem has been solved. Thanks sjhalayka!!