take a look at the code below.
i used this way : firstly i found the smallest blob's area (minimum_area
)
and later used contourArea / minimum_area
for each blob to increase total blobs count.
(it is not perfect but just to show a way you can improve this approach for better results)
#include <opencv2/highgui.hpp>
#include <opencv2/imgproc.hpp>
using namespace cv;
using namespace std;
int main( int argc, const char** argv )
{
Mat img = imread(argv[1]);
if(img.empty())
{
return -1;
}
vector<vector<Point> > contours;
Mat bw,bgr[3];
split( img,bgr );
int red_blobs_count = 0;
cvtColor(bgr[1],bw,COLOR_GRAY2BGR);
bw = img-bw;
cvtColor(bw,bw,COLOR_BGR2GRAY);
bw = bw > 10;
findContours( bw, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE );
int minimum_area = img.cols * img.rows;
for( size_t i = 0; i< contours.size(); i++ )
{
int _contourArea = contourArea(contours[i]);
if( _contourArea > 10 & minimum_area > _contourArea )
{
minimum_area = _contourArea ;
}
}
for( size_t i = 0; i< contours.size(); i++ )
{
int _contourArea = contourArea(contours[i]);
if( _contourArea > 10 )
{
Rect minRect = boundingRect( Mat(contours[i]) );
rectangle(img,minRect,Scalar(0,255,0));
red_blobs_count += _contourArea / minimum_area;
putText(img, format("%.02f",(float)_contourArea / minimum_area), minRect.tl(),FONT_HERSHEY_SIMPLEX, 0.5, Scalar(255,255,0), 2);
putText(img, format("%d",red_blobs_count), minRect.br(),FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0,127,255), 2);
}
}
putText(img, format("red_blobs_count = %d",red_blobs_count), Point(50, 30),FONT_HERSHEY_SIMPLEX, 0.8, Scalar(0,255,0), 2);
imshow( "red_blobs_count", img );
waitKey(0);
return 0;
}
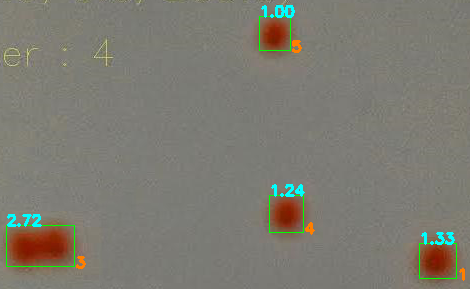
you can check results of this image. you can see some groups not calculated right but you can improve calculation algorithm.
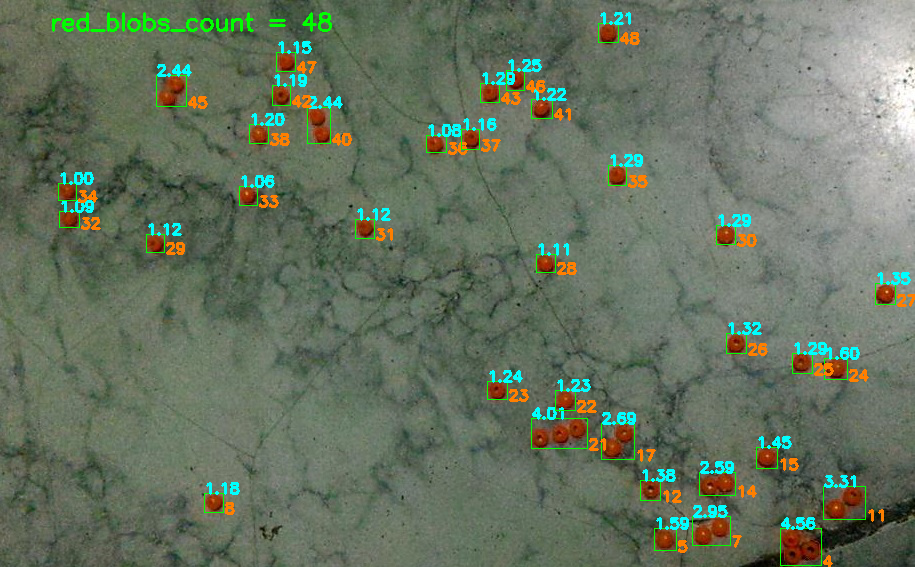
maybe this will help. or if your blob objects are nearly same size you can check area of blobs.
Thanks for answer sir, but how when try in android sir ? in my project just detect for HSV color sir, how when from size of object sir ?
could you post your whole source code if possible.
but its so many sir, no problem ? or i send to your email ?
you can send by e-mail but sorry i am not familiar with java.if you can convert it i can try to implement a c++ code.
your email sir ? maybe i'll try sir.
my username @hotmail.com
alright sir, maybe if not familiar in java you can suggest me which part i must modification ,i've done send to your email sir.