I would do a simple threshold on the image. Then use cv::findContours()
an choose the correct contours with cv::contourArea(
). After that you can cut out the region with creating a rectangle around it (cv::boundingRect
) and copy the specific part to a new cv::Mat
.
Perhabs you have to dilate the image before, if the small bright regions are not seperated from the large ones by cv::findContours()
. Also you can make the size of the bounding rectangle a bit larger if you want.
sample c++ code below can easily be converted to Python
#include "opencv2/highgui/highgui.hpp"
#include "opencv2/imgproc/imgproc.hpp"
#include <iostream>
using namespace cv;
using namespace std;
int main( int, char** argv )
{
/// Load source image
Mat src = imread(argv[1]);
if (src.empty())
{
cerr << "No image supplied ..." << endl;
return -1;
}
/// Convert image to gray
Mat src_gray;
cvtColor( src, src_gray, COLOR_BGR2GRAY );
threshold( src_gray, src_gray, 250, 255, THRESH_BINARY );
//erode( src_gray, src_gray, Mat());
/// Find contours
vector<vector<Point> > contours;
findContours( src_gray, contours, RETR_TREE, CHAIN_APPROX_SIMPLE );
/// Draw contours
for( size_t i = 0; i< contours.size(); i++ )
{
Scalar color = Scalar( 0, 0, 255 );
Rect _boundingRect = boundingRect( contours[i] );
if( _boundingRect.height < 50 )
drawContours( src, contours, (int)i, color, 2 );
}
/// Show in a window
imshow( "Contours", src );
waitKey(0);
return(0);
}
after threshold you will get this binarized image
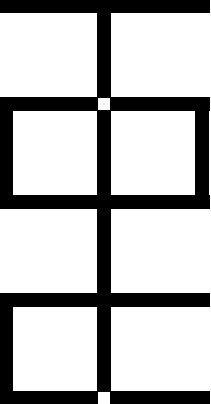
finally you will get your desired parts by contour analyzing
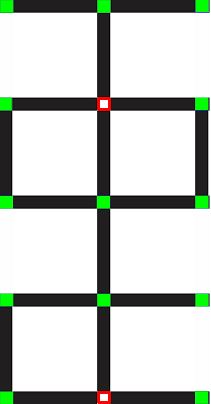
@matman could you post your comment as an answer. i will add a sample code in your answer. i was just trying exactly the same method you explained.