Here's one solution.
Do a binary inverse threshold so the letters are white and background is dark.
Use findContours function with the RETR_EXTERNAL setting to get a contour for each of the 8 letters.
Sort these contours by their x value so that they are in order left to right.
Do this for 2 different font images so that you have 2 sets of 8 contours.
For each contour pair, get their boundingRects.
Extract the 2 roi's and resize one if they are not the same size.
Then use template matching to compare the two roi's (I used TM_CCOEFF_NORMED).
In the match or score image find the max score with minMaxLoc
Notice the scores below are much higher for pairs from the same font than for a different font.
vector<vector<Point>> srcContours;
vector<vector<Point>> src2Contours;
Mat templImg, testImg;
Mat scoreImg;
double maxScore;
Mat templClrImg, testClrImg;
Mat compImg;
findContours(srcImg, srcContours, RETR_EXTERNAL, CHAIN_APPROX_NONE);
findContours(src2Img, src2Contours, RETR_EXTERNAL, CHAIN_APPROX_NONE);
sort(srcContours.begin(), srcContours.end(), [](const vector<Point>& a, const vector<Point>& b) {
Point2f actr = ContourFindCenter(a);
Point2f bctr = ContourFindCenter(b);
return actr.x < bctr.x;
});
sort(src2Contours.begin(), src2Contours.end(), [](const vector<Point>& a, const vector<Point>& b) {
Point2f actr = ContourFindCenter(a);
Point2f bctr = ContourFindCenter(b);
return actr.x < bctr.x;
});
for (int i = 0; i < srcContours.size(); ++i) {
Rect rect = boundingRect(srcContours[i]);
Rect rect2 = boundingRect(src2Contours[i]);
templImg = Mat(srcImg, rect);
testImg = Mat(src2Img, rect2);
if (rect != rect2) {
resize(testImg, testImg, rect.size());
}
matchTemplate(testImg, templImg, scoreImg, TM_CCOEFF_NORMED);
minMaxLoc(scoreImg, 0, &maxScore, 0, 0);
}
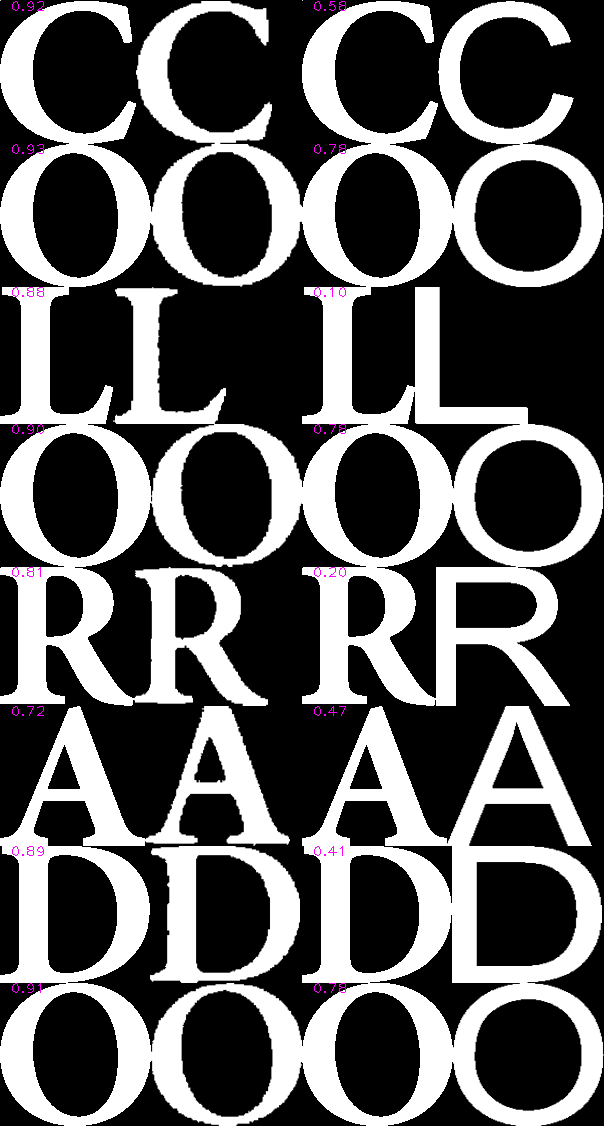
define similar.
@berak, image 2 and 3 have font Adobe Arabic Bold hence image 2 and 3 are similar . From the given figure we can infer that image 1 does not match in font to image 3. Hence image 1 and image 3 are not similar