let's try to build a most simple one from scratch ;)
step 1
create a new android application project, and add the opencv libraries to it.
( you have the opencv project in your workspace, do you ? )
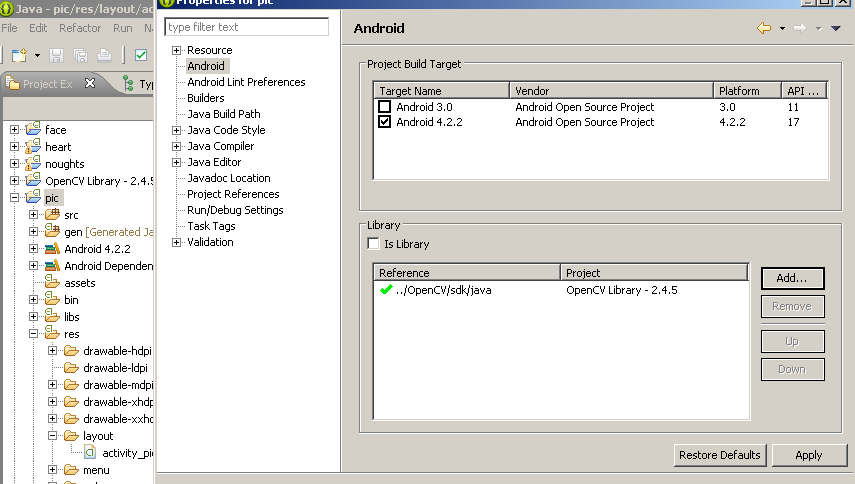
step2
we'll need an imageview to show our image:
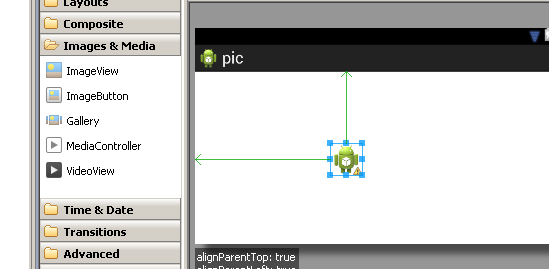
step3
get your hands dirty with code ;)
package com.example.pic;
import org.opencv.android.BaseLoaderCallback;
import org.opencv.android.LoaderCallbackInterface;
import org.opencv.android.OpenCVLoader;
import org.opencv.android.Utils;
import org.opencv.core.Core;
import org.opencv.core.CvType;
import org.opencv.core.Mat;
import org.opencv.core.Point;
import org.opencv.core.Scalar;
import android.os.Bundle;
import android.widget.ImageView;
import android.app.Activity;
import android.graphics.Bitmap;
public class PicActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_pic);
}
private BaseLoaderCallback mLoaderCallback = new BaseLoaderCallback(this) {
@Override
public void onManagerConnected(int status) {
if (status == LoaderCallbackInterface.SUCCESS ) {
// now we can call opencv code !
helloworld();
} else {
super.onManagerConnected(status);
}
}
};
@Override
public void onResume() {;
super.onResume();
OpenCVLoader.initAsync(OpenCVLoader.OPENCV_VERSION_2_4_5,this, mLoaderCallback);
// you may be tempted, to do something here, but it's *async*, and may take some time,
// so any opencv call here will lead to unresolved native errors.
}
public void helloworld() {
// make a mat and draw something
Mat m = Mat.zeros(100,400, CvType.CV_8UC3);
Core.putText(m, "hi there ;)", new Point(30,80), Core.FONT_HERSHEY_SCRIPT_SIMPLEX, 2.2, new Scalar(200,200,0),2);
// convert to bitmap:
Bitmap bm = Bitmap.createBitmap(m.cols(), m.rows(),Bitmap.Config.ARGB_8888);
Utils.matToBitmap(m, bm);
// find the imageview and draw it!
ImageView iv = (ImageView) findViewById(R.id.imageView1);
iv.setImageBitmap(bm);
}
}