Hi @Nirmalan!
Here is my Simple algorithm to smooth with Mask!
bool MaskedSmooth(const Mat mSrc,const Mat mMask,Mat &mDst, double Sigma, double MaskRadius)
{
if(mSrc.empty())
{
return 0;
}
if(MaskRadius<1)
MaskRadius=1.0;
if(Sigma<1)
Sigma=1.0;
Mat mGSmooth;
mDst = Mat(mSrc.size(),mSrc.type());
GaussianBlur(mSrc,mGSmooth,Size(0,0),Sigma); //You can add any Smoothing algorithm here e.g Median, Billateral, etc...
GaussianBlur(mMask,mMask,Size(0,0),MaskRadius);
for (size_t Rows = 0; Rows < mSrc.rows; Rows++)
{
for (size_t Cols = 0; Cols < mSrc.cols; Cols++)
{
Vec3b pSrc = mSrc.at<Vec3b>(Rows, Cols);
Vec3b pSSrc = mGSmooth.at<Vec3b>(Rows, Cols);
Vec3b &pDest = mDst.at<Vec3b>(Rows, Cols);
uchar Mask = mMask.at<uchar>(Rows, Cols);
float Percent = Mask / 255.0;
pDest = (1 - Percent)* pSrc + (pSSrc * Percent);
}
}
return true;
}
bool MaskedSmoothOptimised(Mat mSrc,Mat mMask,Mat &mDst, double Sigma, double MaskRadius)
{
if(mSrc.empty())
{
return 0;
}
if(MaskRadius<1)
MaskRadius=1.0;
if(Sigma<1)
Sigma=1.0;
Mat mGSmooth;
cvtColor(mMask,mMask,COLOR_GRAY2BGR);
mDst = Mat(mSrc.size(),CV_32FC3);
mMask.convertTo(mMask,CV_32FC3,1.0/255.0);
mSrc.convertTo(mSrc,CV_32FC3,1.0/255.0);
GaussianBlur(mSrc,mGSmooth,Size(0,0),Sigma);
GaussianBlur(mMask,mMask,Size(0,0),MaskRadius);
Mat M1,M2,M3;
subtract(Scalar::all(1.0),mMask,M1);
multiply(M1,mSrc,M2);
multiply(mMask,mGSmooth,M3);
add(M2,M3,mDst);
return true;
}
int _tmain(int argc, _TCHAR* argv[])
{
string sSFileNameEx = "Test.png";
string sMFileNameEx = "Test_mask.bmp";
Mat mSrc, mSrc_Mask,mResult;
mSrc = imread(sSFileNameEx, 1);
mSrc_Mask = imread(sMFileNameEx, 0);
if (mSrc_Mask.empty() || mSrc.empty())
{
cout << "[Error]! Invalid Input Image";
return 0;
}
imshow("mSrc", mSrc);
imshow("mSrc_Gray", mSrc_Mask);
MaskedSmooth(mSrc,mSrc_Mask,mResult,5.0,25.0);
imshow("Masked Smooth Results", mResult);
waitKey(0);
return 0;
}
Source Image:
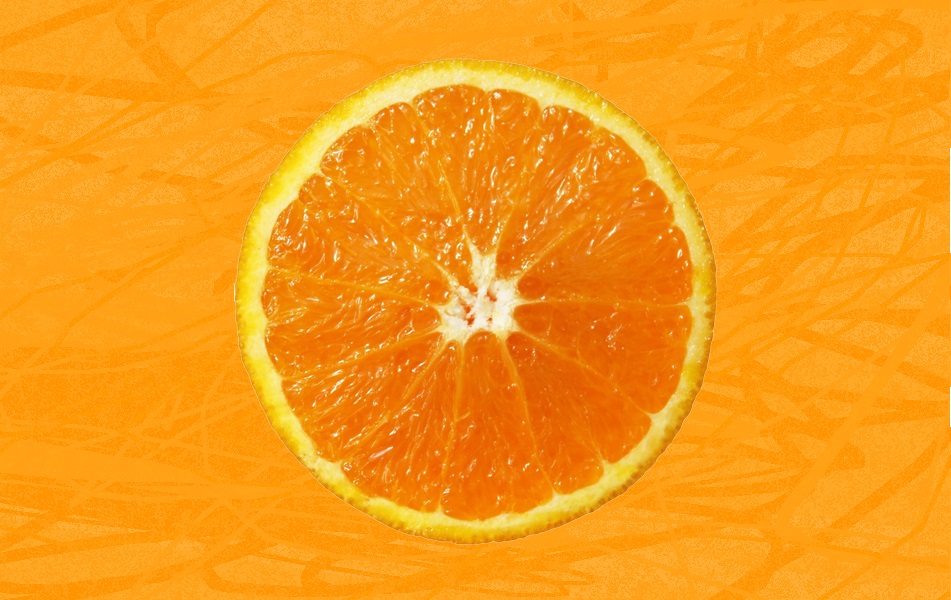
Mask:
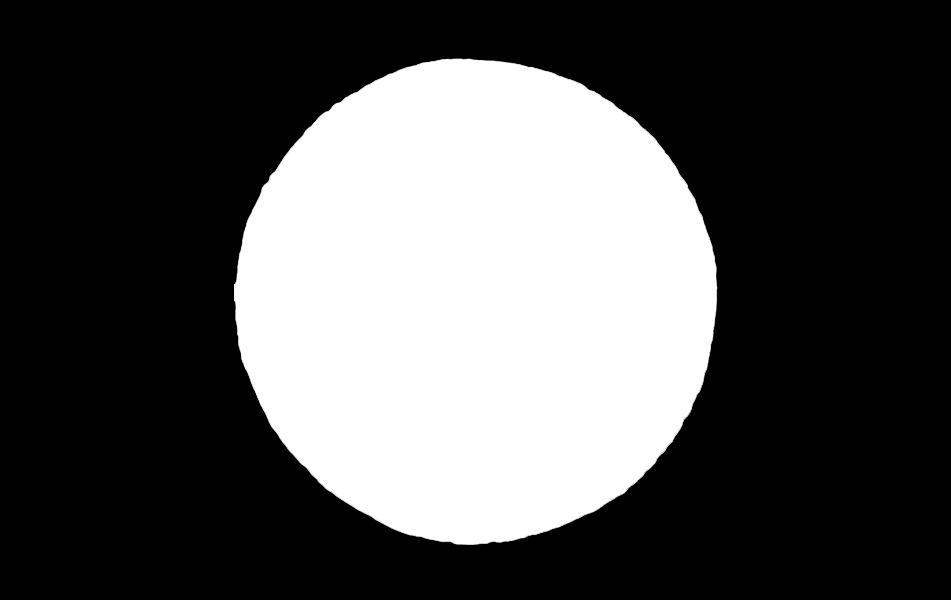
Source Smoothed
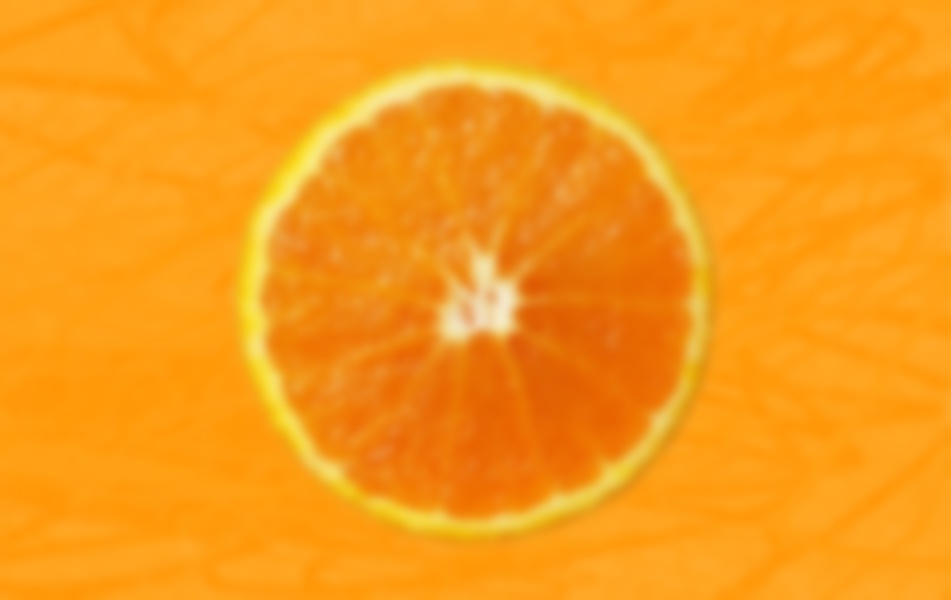
Mask Smoothed
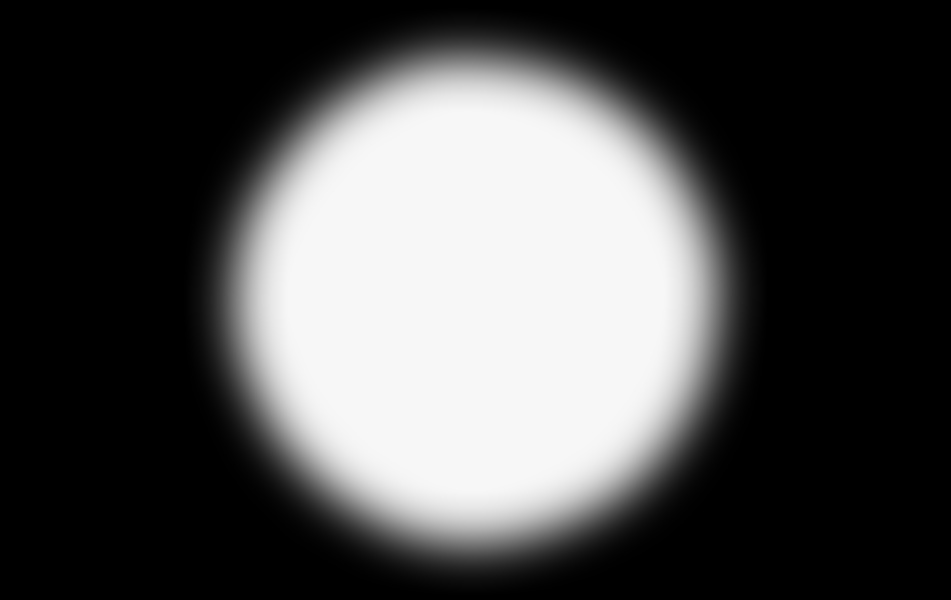
Output Masked Smoothed:
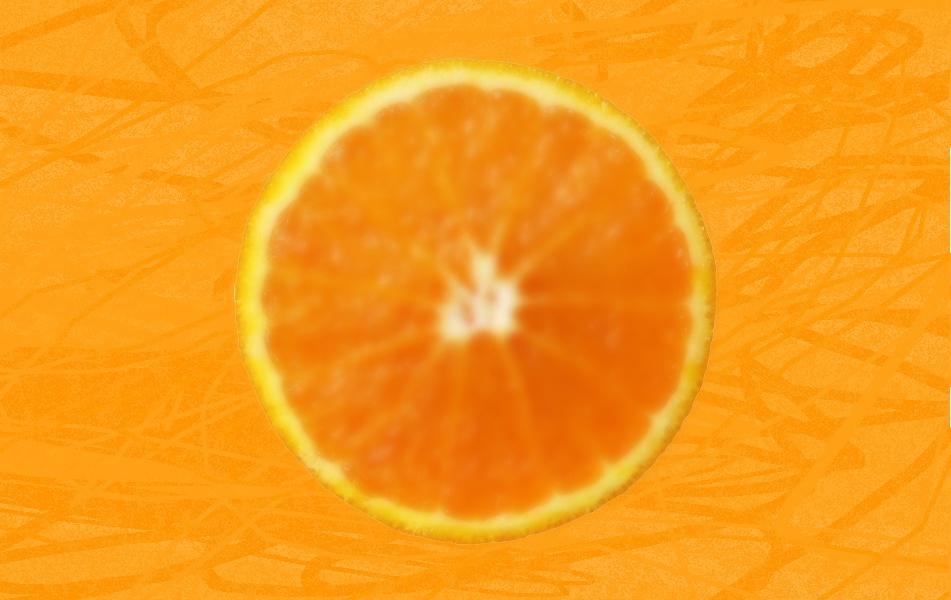