EXC_BAD_ACCESS while Calculating Descriptor
I try to calculate a SIFT Descriptor out of KeyPoints found by the FAST Corner Detection. For that, I adapted some functions from the Sift.cpp and changed them so they can be used with FAST generated KeyPoints with nonmaxsuppression. While I try to calculate the Descriptor I get an EXC_BAD_ACCESS Error and I cant find the origin of this Error. I tried to make a example as small as possible of my code where the Error is generated with no constants or aliases. When you want to know more about the full code see my Git or look at this Question where already some things have been explained in the comments. You can get the Example Image with this Link Now the code:
#include <opencv2/opencv.hpp>
#include <opencv2/core/hal/hal.hpp>
using namespace cv;
int descriptorSize() {
// Descr_Width * Descr_Width * Descr_hist_bins
return 3*3*4;
}
static void calcSiftDescriptor( const Mat& img, Point2f ptf, float ori, float scl,
int d, int n, float* dst )
{
Point pt(cvRound(ptf.x), cvRound(ptf.y));
float cos_t = cosf(ori*(float)(CV_PI/180));
float sin_t = sinf(ori*(float)(CV_PI/180));
float bins_per_rad = n / 360.f;
float exp_scale = -1.f/(d * d * 0.5f);
// 3.f = Descr_scale_factor
float hist_width = 3.f * scl;
int radius = cvRound(hist_width * 1.4142135623730951f * (d + 1) * 0.5f);
// Clip the radius to the diagonal of the image to avoid autobuffer too large exception
radius = std::min(radius, (int) sqrt(((double) img.cols)*img.cols + ((double) img.rows)*img.rows));
cos_t /= hist_width;
sin_t /= hist_width;
int i, j, k, len = (radius*2+1)*(radius*2+1), histlen = (d+2)*(d+2)*(n+2);
int rows = img.rows, cols = img.cols;
AutoBuffer<float> buf(len*6 + histlen);
float *X = buf, *Y = X + len, *Mag = Y, *Ori = Mag + len, *W = Ori + len;
float *RBin = W + len, *CBin = RBin + len, *hist = CBin + len;
for( i = 0; i < d+2; i++ )
{
for( j = 0; j < d+2; j++ )
for( k = 0; k < n+2; k++ )
hist[(i*(d+2) + j)*(n+2) + k] = 0.;
}
for( i = -radius, k = 0; i <= radius; i++ )
for( j = -radius; j <= radius; j++ )
{
// Calculate sample's histogram array coords rotated relative to ori.
// Subtract 0.5 so samples that fall e.g. in the center of row 1 (i.e.
// r_rot = 1.5) have full weight placed in row 1 after interpolation.
float c_rot = j * cos_t - i * sin_t;
float r_rot = j * sin_t + i * cos_t;
float rbin = r_rot + d/2 - 0.5f;
float cbin = c_rot + d/2 - 0.5f;
int r = pt.y + i, c = pt.x + j;
if( rbin > -1 && rbin < d && cbin > -1 && cbin < d &&
r > 0 && r < rows - 1 && c > 0 && c < cols - 1 )
{
float dx = (float)(img.at<float>(r, c+1) - img.at<float>(r, c-1));
float dy = (float)(img.at<float>(r-1, c) - img.at<float>(r+1, c));
X[k] = dx; Y[k] = dy; RBin[k] = rbin; CBin[k] = cbin;
W[k] = (c_rot * c_rot + r_rot * r_rot)*exp_scale;
k++;
}
}
len = k;
cv::hal::fastAtan2(Y, X, Ori ...
can we have your image, too ?
-2147483648 = 0x80000000 (which looks more like some "mark this invalid", than a number)
are you able to debug it ?
Totally forgot the Image Sorry. I uploaded it here I can run my Example in XCode and get the Error at line 111
hist[idx] += v_rco000;
like before in the big Project.ok, i can reproduce it, v_rco000 is NaN at idx=88
(win10, x64, mingw64)
Checked that Again. One step before I get the Error, I also have, that all Values of
v_[...]
are NaN atidx = 15
the variableo0 = 3
. After that, again all values are NaN and i have the out of boundsidx
occuring becauseo0
is also out of bounds. So the Error should be because of the Calculation ofMag, Ori, X and Y
?CV_Assert( ! cvIsNaN(mag) );
<-- i guess, you'll need a lot of similar assertions, and slowly work your way up the call stackok, I'll try that, but I'm not very familiar with Assertions. Should I set that after The loop where mag is filled? And similar assertions I think you mean, that I try that also with the other Pointers?
in my case,
float mag = Mag[k]*W[k];
is already NaN. so you need to check Mag and Wyes, try some other vars, too !
So after setting the Assertion I see that
mag = NaN
whenk = 8
. After that I testet which one of the two Matrix variables isNaN
which isMag[k]
. EvenOri[k]
does not throw a Assertion Error before that code. So it seems, thatMag
is the problem then?Also set Assertions at the calculation of
dx and dy
now. Both also throw a Assertiondy = NaN
Wherec = 363
andr = 103
anddx = NaN
wherec = 362
andr=103
normally, both are still in the Image bounds which are 724x724 so I don't have a clue, why they areNaN
in addition, they both dont seem to throw the Assertion at the Point whereMag
would beNaN
.just to cheer you up in the meantime: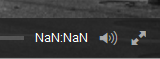